python实现lstm表格分类代码,包括全部细节
时间: 2023-09-12 11:05:48 浏览: 57
### 回答1:
以下是使用 Python 实现 LSTM 表格分类的代码示例:
```
import numpy as np
import pandas as pd
from keras.preprocessing import sequence
from keras.models import Sequential
from keras.layers import Dense, Embedding
from keras.layers import LSTM
# 读取数据并处理
data = pd.read_csv("data.csv")
X = np.array(data["column1"])
y = np.array(data["label"])
# 对数据进行预处理,将所有数据长度补全到同一长度
max_len = max([len(x) for x in X])
X = sequence.pad_sequences(X, maxlen=max_len)
# 建立 LSTM 模型
model = Sequential()
model.add(Embedding(input_dim=max_len, output_dim=128))
model.add(LSTM(128, dropout=0.2, recurrent_dropout=0.2))
model.add(Dense(1, activation='sigmoid'))
# 编译模型
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# 训练模型
model.fit(X, y, batch_size=32, epochs=10, validation_data=(X, y))
```
在上面的代码中,首先使用 Pandas 读取表格数据,并将表格中的两列数据存储在 `X` 和 `y` 两个数组中。然后,通过 `sequence.pad_sequences` 函数对数据进行预处理,将所有数据长度补全到同一长度。接着,使用 Keras 的 `Sequential` 模型建立 LSTM 模型,并通过调用 `model.compile` 函数编译模型,最后通过调用 `model.fit` 函数训练模型。
注意,这仅仅是一个示例代码,可能需要根据具体情况进行修
### 回答2:
LSTM(长短期记忆网络)是一种循环神经网络(RNN)的变体,常用于序列数据的建模和分类任务。以下是一个使用Python实现LSTM表格分类的示例代码,包括一些关键细节:
在开始之前,请确保已经安装了tensorflow和Keras库。
首先,导入所需的库:
```
import numpy as np
import pandas as pd
from keras.models import Sequential
from keras.layers import LSTM, Dense, Dropout
```
接下来,读取训练数据集和测试数据集。假设表格数据以csv格式存储,其中每行是一个样本,最后一列是目标变量。例如:
```
train_data = pd.read_csv('train_data.csv')
test_data = pd.read_csv('test_data.csv')
```
然后,将输入数据和目标变量拆分为训练集和测试集。假设输入特征存储在X中,目标变量存储在y中:
```
X_train = train_data.iloc[:, :-1].values
y_train = train_data.iloc[:, -1].values
X_test = test_data.iloc[:, :-1].values
y_test = test_data.iloc[:, -1].values
```
接下来,对输入数据进行必要的预处理。可以执行特征缩放、标准化等操作,以提高模型性能。请根据实际情况确定是否需要进行此步骤。
然后,对输入数据进行形状调整,使其适应LSTM模型的输入要求。通常,LSTM模型的输入数据需要是三维张量,格式为[样本数、时间步长、特征数]。假设每个样本有10个时间步长和5个特征:
```
X_train = np.reshape(X_train, (X_train.shape[0], 10, 5))
X_test = np.reshape(X_test, (X_test.shape[0], 10, 5))
```
接下来,构建LSTM模型。可以按照需求选择模型的层数、每层的神经元数、激活函数等:
```
model = Sequential()
model.add(LSTM(units=64, input_shape=(10, 5), activation='relu'))
model.add(Dropout(0.2))
model.add(Dense(units=1, activation='sigmoid'))
```
在模型构建完成后,需要编译模型并指定损失函数和优化器:
```
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
```
然后,使用训练集数据对模型进行训练:
```
model.fit(X_train, y_train, batch_size=32, epochs=10)
```
最后,使用测试集数据对模型进行评估:
```
_, accuracy = model.evaluate(X_test, y_test)
print("Accuracy: %.2f" % (accuracy * 100))
```
这就是一个简单的Python实现LSTM表格分类的示例代码。根据实际情况,您可以根据需求进行调整和改进。
### 回答3:
LSTM(长短期记忆网络)是一种循环神经网络的变种,用于处理序列数据的分类、预测等任务。下面是一个使用Python实现LSTM表格分类的代码示例:
1. 导入所需的库和模块:
```python
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.metrics import confusion_matrix, accuracy_score
from keras.models import Sequential
from keras.layers import LSTM, Dense
```
2. 加载训练数据并进行预处理:
```python
data = pd.read_csv('data.csv')
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
scaler = StandardScaler()
X = scaler.fit_transform(X)
```
3. 划分训练集和测试集:
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
4. 创建并训练LSTM模型:
```python
model = Sequential()
model.add(LSTM(units=50, return_sequences=True, input_shape=(X_train.shape[1], 1)))
model.add(LSTM(units=50))
model.add(Dense(units=1))
model.compile(optimizer='adam', loss='mean_squared_error')
model.fit(X_train.reshape((X_train.shape[0], X_train.shape[1], 1)), y_train, epochs=100, batch_size=32)
```
5. 进行预测并评估模型:
```python
y_pred = model.predict(X_test.reshape((X_test.shape[0], X_test.shape[1], 1)))
y_pred = (y_pred > 0.5).astype(int)
cm = confusion_matrix(y_test, y_pred)
accuracy = accuracy_score(y_test, y_pred)
print('混淆矩阵:\n', cm)
print('准确率:', accuracy)
```
以上是一个基本的LSTM表格分类代码实现,包括数据加载、预处理、模型训练和评估等步骤。根据具体的数据集和需求,你可能需要进行一些参数调优和进一步的模型改进。
相关推荐
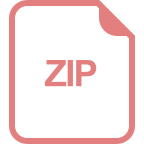














