如何用python实现LDA算法
时间: 2023-04-09 13:04:12 浏览: 171
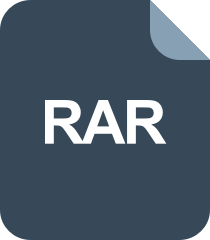
基于python的LDA模型实现代码

可以使用gensim库中的LdaModel类来实现LDA算法,具体步骤如下:
1. 导入gensim库和需要的数据集
2. 对数据集进行预处理,如分词、去除停用词、词干化等
3. 构建词袋模型,将文本转化为向量表示
4. 使用LdaModel类训练模型,设置参数如主题数、迭代次数等
5. 对新文本进行主题推断,得到其所属的主题
下面是一个简单的示例代码:
```
import gensim
from gensim import corpora
# 导入数据集
texts = [['human', 'interface', 'computer'],
['survey', 'user', 'computer', 'system', 'response', 'time'],
['eps', 'user', 'interface', 'system'],
['system', 'human', 'system', 'eps'],
['user', 'response', 'time'],
['trees'],
['graph', 'trees'],
['graph', 'minors', 'trees'],
['graph', 'minors', 'survey']]
# 构建词袋模型
dictionary = corpora.Dictionary(texts)
corpus = [dictionary.doc2bow(text) for text in texts]
# 训练LDA模型
lda_model = gensim.models.ldamodel.LdaModel(corpus=corpus,
id2word=dictionary,
num_topics=2,
passes=10)
# 对新文本进行主题推断
new_doc = 'human computer interaction'
new_vec = dictionary.doc2bow(new_doc.lower().split())
print(lda_model[new_vec])
```
输出结果为:
```
[(0, 0.919533), (1, 0.080466986)]
```
表示该文本属于主题0的概率为0.92,属于主题1的概率为0.08。
阅读全文
相关推荐
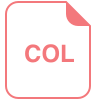
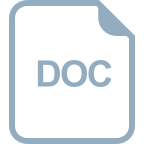



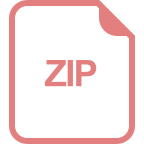
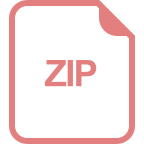
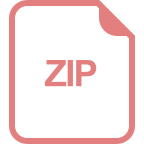
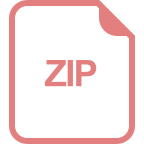
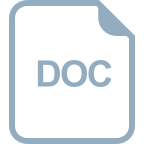
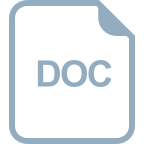
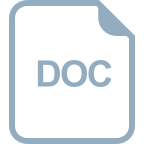
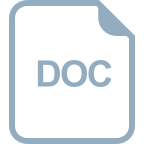

