stm32通过PWM实现多个呼吸灯的具体代码实现
时间: 2024-10-12 19:07:37 浏览: 56
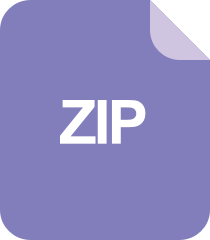
STM32 PWM波实现全彩呼吸灯
STM32通过PWM实现多个呼吸灯的代码实现通常需要以下几个步骤:
首先,你需要配置好GPIO作为输出,连接到每个LED灯,并初始化PWM模块。这里假设你使用的是定时器TIMx。
```c
#include "stm32f4xx_hal.h"
// LED和GPIO定义
#define LED_1 GPIO_PIN_0
#define LED_2 GPIO_PIN_1
// PWM设置相关的宏
#define PWM_CHANNEL 1 // TIMx的Channel 1 (可以根据实际情况调整)
#define PWM_PERIOD 5000 // 5ms周期
void ledSetup(void) {
GPIO_InitTypeDef GPIO_InitStruct;
HAL_TIM_PWM_Init(&htim1);
GPIO_InitStruct.Pin = LED_1 | LED_2; // 连接所有LED
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
}
```
然后,你可以创建一个函数来设置PWM的频率和 duty cycle(占空比),并用于LED的呼吸效果。
```c
void setPwmDutyCycle(uint8_t led, uint16_t dutyCycle) {
TIM_OC_InitTypeDef ocInitStructure;
ocInitStructure.Channel = PWM_CHANNEL;
ocInitStructure.Ocn = 0; // 无捕获
ocInitStructure.Pulse = dutyCycle;
ocInitStructure.Mode = TIM_OCMODE_PWM1; // 输出模式
ocInitStructure.OCPolarity = TIM_OCPOLARITY_HIGH; // 高电平占空比
ocInitStructure.OCDRB = 0; // 分频因子为1
if (led == 1) {
htim1.OCR1n = dutyCycle; // 更新LED1的duty cycle
} else {
htim1.OCR2n = dutyCycle; // 更新LED2的duty cycle
}
HAL_TIM_PWM_ConfigChannel(&htim1, &ocInitStructure);
HAL_TIM_PWM_Start(&htim1, TIM_CHANNEL_1); // 启动对应的PWM通道
}
```
接下来编写呼吸灯的主循环函数,改变duty cycle来模拟灯光的闪烁效果。
```c
void breathingLight(uint16_t period, uint16_t fadeSteps) {
uint16_t currentFadeStep = 0;
while (1) {
for (currentFadeStep = 0; currentFadeStep <= fadeSteps; ++currentFadeStep) {
float dutyRatio = currentFadeStep * ((float)PWM_PERIOD / fadeSteps);
setPwmDutyCycle(1, PWM_PERIOD - dutyRatio); // LED 1 从0%到100%
setPwmDutyCycle(2, dutyRatio); // LED 2 从0%到100%
HAL_Delay(period);
}
// 反向过程,从100%减至0%
for (currentFadeStep = fadeSteps; currentFadeStep >= 0; --currentFadeStep) {
float dutyRatio = currentFadeStep * ((float)PWM_PERIOD / fadeSteps);
setPwmDutyCycle(1, PWM_PERIOD - dutyRatio); // LED 1 从100%到0%
setPwmDutyCycle(2, dutyRatio); // LED 2 从100%到0%
HAL_Delay(period);
}
}
}
```
最后,在`main()`中启动上述功能:
```c
int main(void) {
ledSetup();
breathingLight(PWM_PERIOD, 10); // 设置呼吸频率和淡入淡出步数
while (1);
return 0;
}
```
阅读全文
相关推荐
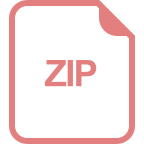
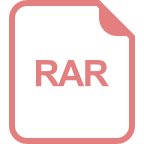
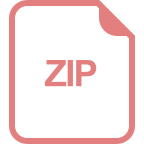
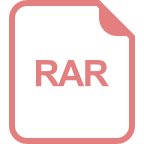
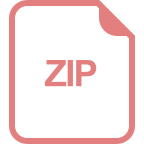
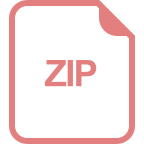
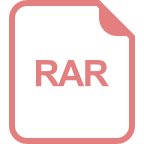
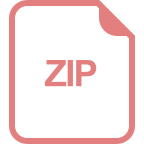
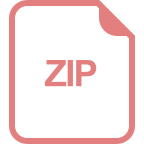
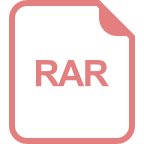
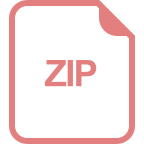
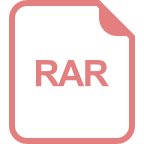
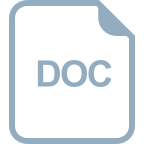
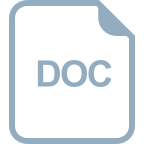
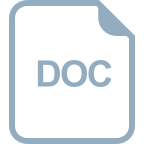
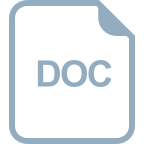

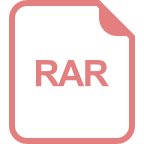
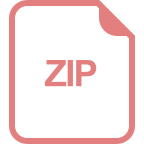