将数值1-10存入链表中,将其逆序输出。c语言写
时间: 2024-10-12 08:08:08 浏览: 16
在C语言中,我们可以使用结构体定义链表节点,并创建一个循环链表来存储数字1到10。然后,我们遍历链表并逐个添加元素,最后再通过递归或迭代的方式将链表逆序。以下是简单的实现步骤:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点并添加数据
Node* addNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 添加元素到链表
void insert(Node** head, int data) {
if (*head == NULL) {
*head = addNode(data);
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = addNode(data);
}
}
// 逆序链表
Node* reverseList(Node* head) {
if (head == NULL || head->next == NULL) {
return head;
}
Node* temp = reverseList(head->next);
head->next->next = head;
head->next = NULL;
return temp;
}
// 输出链表
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
Node* head = NULL; // 初始化链表头指针
for (int i = 1; i <= 10; i++) {
insert(&head, i); // 依次插入数字
}
// 逆序链表并打印结果
head = reverseList(head);
printList(head);
return 0;
}
```
运行这个程序,你会看到从10到1的数字按照逆序顺序输出。
阅读全文
相关推荐
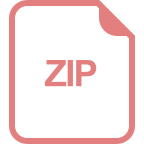
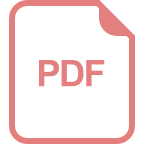
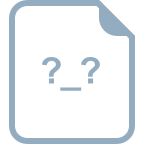















