java 读取usb扫码台
时间: 2023-10-25 08:03:53 浏览: 41
Java可以通过使用Java的串口通信库来读取USB扫码台。要读取USB扫码台,首先需要识别并打开与USB扫码台连接的串口。
第一步是使用Java的串口通信库来扫描可用的串口端口,并找到与USB扫码台连接的端口。可以使用`CommPortIdentifier`类来实现这个功能。此类提供了一个静态方法`getPortIdentifiers()`,它返回所有可用的串口端口的枚举。
第二步是识别出与USB扫码台连接的串口。可以通过遍历可用的串口端口并检查其类型来实现。当找到一个与USB扫码台连接的串口时,可以使用`CommPortIdentifier`的方法`open()`来打开该串口。
第三步是通过打开的串口获取输入流,以便从USB扫码台读取数据。可以使用`CommPort`接口的`getInputStream()`方法来实现。该方法返回一个`InputStream`对象,可以使用此对象读取从USB扫码台发送的数据。
最后一步是读取扫码台发送的数据。可以通过使用`InputStream`的`read()`方法来实现。此方法将读取一个字节,并返回读取的字节的值。
以上是使用Java读取USB扫码台的基本步骤。要完善这个过程,可能需要处理异常、设置数据传输的格式等。此外,具体实现可能因不同的USB扫码台而有所差异。在实际应用中,还需要根据具体的USB扫码台型号和连接接口进行相应的调整和配置。
相关问题
labview读取usb扫码枪
对于使用LabVIEW读取USB扫码枪的操作,以下是一个基本的步骤:
1. 首先,插入USB扫码枪到计算机的USB接口上。这个时候,计算机应该会自动安装扫码枪所需的驱动程序。
2. 打开LabVIEW开发环境,在项目中创建一个新的VI(虚拟设备界面)。
3. 在VI中,使用LabVIEW提供的“VISA”函数库来创建一个VISA(虚拟设备)对象。VISA是一种功能强大的通信协议,用于与外部设备进行通信。
4. 使用VISA函数库中的函数,通过选择正确的端口号和设备地址,将VISA对象与扫码枪进行连接。这通常包括确认扫码枪所连接到的USB端口号。
5. 建立与扫码枪的通信,并配置扫码枪的相关参数。这包括设置扫码模式(比如连续扫描或手动触发扫描),设置扫码枪为等待模式等等。
6. 在LabVIEW的代码中,使用VISA函数库提供的读取和写入功能,发送命令给扫码枪以开始扫描或者从扫描枪中读取扫描结果。
7. 根据需要,可以在LabVIEW中进行一些额外的处理,如解析扫描结果、处理异常情况等。
总的来说,通过使用VISA函数库和一些基本的LabVIEW编程技巧,可以相对容易地实现LabVIEW与USB扫码枪的连接和通信。这样,你就可以在LabVIEW中读取扫码枪的扫描结果,并将其用于后续的数据处理和分析。
单片机读取USB扫码枪数据
要实现单片机读取USB扫码枪数据,可以通过以下步骤:
1. 确认扫码枪支持USB接口,并且可以通过USB接口发送数据。
2. 在单片机上连接USB转串口模块,将USB接口转化为串口接口。
3. 在单片机上编写串口接收程序,通过串口接收USB转串口模块发送的数据。
4. 根据扫码枪的数据格式,解析接收到的数据,获取扫码枪扫描的条形码或二维码信息。
需要注意的是,不同的扫码枪可能有不同的数据格式和通信协议,因此需要根据具体的扫码枪型号来编写相应的程序。
相关推荐
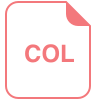











