c语言已知无向图G=(V,E),给出求图G的连通分量个数的算法
时间: 2024-04-16 08:26:21 浏览: 122
你可以使用深度优先搜索(DFS)算法来求解图G的连通分量个数。下面是一个基于DFS的算法:
1. 创建一个布尔型数组visited,用于标记顶点是否被访问过。初始化visited数组为false。
2. 初始化连通分量个数count为0。
3. 对于图中的每个顶点v,如果v尚未被访问过,则执行以下步骤:
a. 通过DFS遍历从v开始的连通分量,并将访问过的顶点标记为已访问。
b. 将count加1,表示发现了一个新的连通分量。
4. 返回count作为连通分量个数。
下面是一个使用C语言实现的示例代码:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_VERTICES 100
// 邻接表节点
typedef struct Node {
int vertex;
struct Node* next;
} Node;
// 图结构
typedef struct Graph {
int numVertices;
Node* adjLists[MAX_VERTICES];
} Graph;
// 创建新节点
Node* createNode(int v) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->vertex = v;
newNode->next = NULL;
return newNode;
}
// 添加边
void addEdge(Graph* graph, int src, int dest) {
// 添加从src到dest的边
Node* newNode = createNode(dest);
newNode->next = graph->adjLists[src];
graph->adjLists[src] = newNode;
// 添加从dest到src的边
newNode = createNode(src);
newNode->next = graph->adjLists[dest];
graph->adjLists[dest] = newNode;
}
// 深度优先搜索
void DFS(Graph* graph, int vertex, bool visited[]) {
visited[vertex] = true;
Node* adjList = graph->adjLists[vertex];
Node* temp = adjList;
while (temp != NULL) {
int connectedVertex = temp->vertex;
if (!visited[connectedVertex]) {
DFS(graph, connectedVertex, visited);
}
temp = temp->next;
}
}
// 求连通分量个数
int findConnectedComponents(Graph* graph) {
bool visited[MAX_VERTICES];
int count = 0;
for (int i = 0; i < graph->numVertices; i++) {
visited[i] = false;
}
for (int i = 0; i < graph->numVertices; i++) {
if (!visited[i]) {
DFS(graph, i, visited);
count++;
}
}
return count;
}
int main() {
Graph* graph = (Graph*)malloc(sizeof(Graph));
graph->numVertices = 6;
for (int i = 0; i < graph->numVertices; i++) {
graph->adjLists[i] = NULL;
}
addEdge(graph, 0, 1);
addEdge(graph, 0, 2);
addEdge(graph, 1, 2);
addEdge(graph, 3, 4);
int connectedComponents = findConnectedComponents(graph);
printf("连通分量个数:%d\n", connectedComponents);
return 0;
}
```
这是一个简单的示例代码,你可以根据需要进行修改和扩展。希望能对你有所帮助!
阅读全文
相关推荐
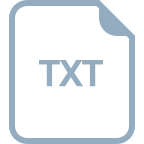






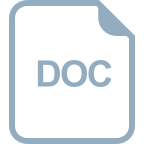
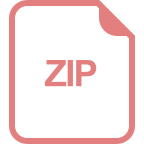
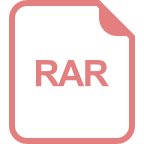
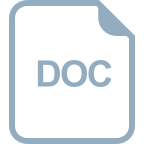
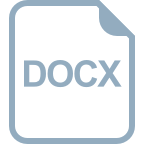
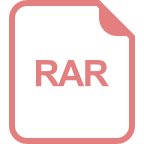
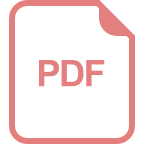
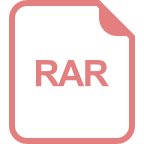
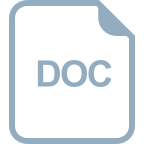
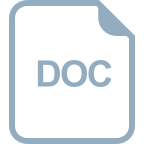