html 鼠标框选表格
时间: 2023-07-05 08:29:14 浏览: 156
要在 HTML 中实现鼠标框选表格,你可以使用 JavaScript 和 CSS。具体实现步骤如下:
1. 在 HTML 中创建一个表格。
2. 使用 CSS 设定表格的样式和外观。
3. 使用 JavaScript 监听鼠标事件,当用户在表格内按下鼠标左键时,记录下鼠标的开始位置。
4. 继续监听鼠标事件,当用户在表格内松开鼠标左键时,记录下鼠标的结束位置。
5. 根据起始位置和结束位置计算出框选区域的位置和大小,将其应用到 CSS 样式中,使其呈现出框选的效果。
以下是一个简单的示例代码,用于实现鼠标框选表格:
```html
<!DOCTYPE html>
<html>
<head>
<style>
table {
border-collapse: collapse;
}
td {
border: 1px solid black;
}
.selection {
background-color: yellow;
}
</style>
<script>
var isSelecting = false;
var startRow, startCol, endRow, endCol;
function handleMouseDown(event) {
isSelecting = true;
startRow = event.target.parentNode.rowIndex;
startCol = event.target.cellIndex;
endRow = startRow;
endCol = startCol;
updateSelection();
}
function handleMouseMove(event) {
if (isSelecting) {
endRow = event.target.parentNode.rowIndex;
endCol = event.target.cellIndex;
updateSelection();
}
}
function handleMouseUp(event) {
isSelecting = false;
}
function updateSelection() {
var table = document.getElementById("myTable");
for (var i = 0; i < table.rows.length; i++) {
for (var j = 0; j < table.rows[i].cells.length; j++) {
var cell = table.rows[i].cells[j];
if (i >= startRow && i <= endRow && j >= startCol && j <= endCol) {
cell.classList.add("selection");
} else {
cell.classList.remove("selection");
}
}
}
}
</script>
</head>
<body>
<table id="myTable">
<tr>
<td>Row 1, Column 1</td>
<td>Row 1, Column 2</td>
<td>Row 1, Column 3</td>
</tr>
<tr>
<td>Row 2, Column 1</td>
<td>Row 2, Column 2</td>
<td>Row 2, Column 3</td>
</tr>
<tr>
<td>Row 3, Column 1</td>
<td>Row 3, Column 2</td>
<td>Row 3, Column 3</td>
</tr>
</table>
<script>
var table = document.getElementById("myTable");
table.addEventListener("mousedown", handleMouseDown);
table.addEventListener("mousemove", handleMouseMove);
table.addEventListener("mouseup", handleMouseUp);
</script>
</body>
</html>
```
在这个示例中,我们创建了一个简单的三行三列的表格,并使用 CSS 设定了表格的样式和外观。我们在 JavaScript 中监听了表格的鼠标事件,并在事件发生时计算出框选区域的位置和大小,然后将其应用到 CSS 样式中,使其呈现出框选的效果。
阅读全文
相关推荐



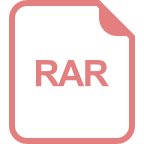









