用c语言写以下代码:为学生数据创建一个栈,学生数据包括配学号、姓名、专业、英语成绩。
时间: 2023-04-12 08:00:34 浏览: 67
以下是用C语言写的代码,用于为学生数据创建一个栈:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct {
int id;
char name[20];
char major[20];
float english_score;
} Student;
typedef struct {
Student data[MAX_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, Student stu) {
if (is_full(s)) {
printf("Stack is full.\n");
return;
}
s->data[++s->top] = stu;
}
Student pop(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty.\n");
exit(1);
}
return s->data[s->top--];
}
int main() {
Stack s;
init(&s);
Student stu1 = { 1, "Tom", "Computer Science", 80.5 };
Student stu2 = { 2, "Jerry", "Mathematics", 90.0 };
Student stu3 = { 3, "Alice", "Physics", 85.5 };
push(&s, stu1);
push(&s, stu2);
push(&s, stu3);
while (!is_empty(&s)) {
Student stu = pop(&s);
printf("ID: %d, Name: %s, Major: %s, English Score: %.1f\n", stu.id, stu.name, stu.major, stu.english_score);
}
return 0;
}
```
这段代码可以创建一个学生数据的栈,包括学号、姓名、专业和英语成绩。可以通过 push 函数将学生数据压入栈中,通过 pop 函数将学生数据从栈中弹出,并打印出学生的信息。
相关推荐
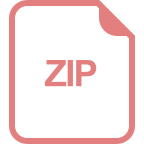
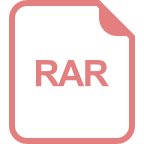














