用java写一个英雄pk界面里面放一个背景图片背景图片,双方有三个英雄可以选择,选择好的英雄的图片放到上面,在选择好的每个图片英雄下面放两个按钮一个是普攻一个是技能,三次普攻之后可以释放一次技能,选择好的英雄图片中间写一个vs两个字。
时间: 2024-01-24 15:17:32 浏览: 10
这是一个基本的Java Swing程序,实现了英雄PK界面的要求。你可以根据需要进行修改和优化。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class HeroPK extends JFrame implements ActionListener {
private JLabel backgroundLabel;
private JLabel hero1Label, hero2Label;
private JButton[] hero1Buttons, hero2Buttons;
private JLabel vsLabel;
private int hero1AttackCount, hero2AttackCount;
public HeroPK() {
// 设置窗口标题
super("英雄PK");
// 设置窗口大小
setSize(800, 600);
// 设置窗口位置
setLocationRelativeTo(null);
// 设置关闭窗口时退出程序
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 设置背景图片
ImageIcon background = new ImageIcon("background.jpg");
backgroundLabel = new JLabel(background);
backgroundLabel.setBounds(0, 0, getWidth(), getHeight());
add(backgroundLabel);
// 添加英雄图片和按钮
hero1Label = new JLabel();
hero1Label.setBounds(50, 50, 150, 200);
backgroundLabel.add(hero1Label);
hero2Label = new JLabel();
hero2Label.setBounds(getWidth() - 200, 50, 150, 200);
backgroundLabel.add(hero2Label);
hero1Buttons = new JButton[2];
hero1Buttons[0] = new JButton("普攻");
hero1Buttons[0].setBounds(50, 300, 100, 50);
hero1Buttons[0].addActionListener(this);
backgroundLabel.add(hero1Buttons[0]);
hero1Buttons[1] = new JButton("技能");
hero1Buttons[1].setBounds(200, 300, 100, 50);
hero1Buttons[1].addActionListener(this);
backgroundLabel.add(hero1Buttons[1]);
hero2Buttons = new JButton[2];
hero2Buttons[0] = new JButton("普攻");
hero2Buttons[0].setBounds(getWidth() - 200, 300, 100, 50);
hero2Buttons[0].addActionListener(this);
backgroundLabel.add(hero2Buttons[0]);
hero2Buttons[1] = new JButton("技能");
hero2Buttons[1].setBounds(getWidth() - 350, 300, 100, 50);
hero2Buttons[1].addActionListener(this);
backgroundLabel.add(hero2Buttons[1]);
// 添加vs标签
vsLabel = new JLabel("VS");
vsLabel.setFont(new Font("Arial", Font.BOLD, 48));
vsLabel.setForeground(Color.WHITE);
vsLabel.setBounds(getWidth() / 2 - 50, getHeight() / 2 - 50, 100, 100);
backgroundLabel.add(vsLabel);
// 显示窗口
setVisible(true);
}
public static void main(String[] args) {
new HeroPK();
}
@Override
public void actionPerformed(ActionEvent e) {
// 判断事件源是哪个按钮
if (e.getSource() == hero1Buttons[0]) {
hero2AttackCount++;
if (hero2AttackCount == 3) {
hero2AttackCount = 0;
System.out.println("英雄2释放技能");
} else {
System.out.println("英雄1普攻");
}
} else if (e.getSource() == hero1Buttons[1]) {
System.out.println("英雄1释放技能");
} else if (e.getSource() == hero2Buttons[0]) {
hero1AttackCount++;
if (hero1AttackCount == 3) {
hero1AttackCount = 0;
System.out.println("英雄1释放技能");
} else {
System.out.println("英雄2普攻");
}
} else if (e.getSource() == hero2Buttons[1]) {
System.out.println("英雄2释放技能");
}
}
}
```
相关推荐
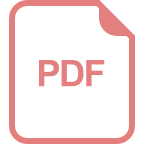
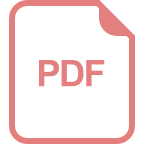
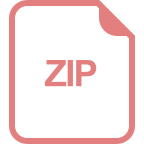














