MediaFile mediaFile = Optional.ofNullable(mediaFileMapper.selectByPrimaryKey(Id)) .orElseThrow(mediaFileMapper.insertAndGetId(mediaFile)),请问写的对吗?
时间: 2024-02-28 10:52:55 浏览: 217
对的,这段代码是有问题的。
首先,`orElseThrow` 方法需要传入一个 `Supplier` 类型的参数,用于在 Optional 对象为空时抛出一个异常。但是,你传入的是一个方法调用 `mediaFileMapper.insertAndGetId(mediaFile)`,这不符合 `Supplier` 的定义。
其次,`mediaFileMapper.insertAndGetId(mediaFile)` 方法没有返回值,因此无法作为 `orElseThrow` 的参数。
如果你想要在 Optional 对象为空时插入数据,可以使用以下代码:
```
MediaFile mediaFile = mediaFileMapper.selectByPrimaryKey(id);
if (mediaFile == null) {
mediaFile = new MediaFile();
mediaFile.setId(id);
mediaFile.setFileName("new file");
mediaFileMapper.insert(mediaFile);
}
```
这样,如果查询不到对应的媒体文件,就会创建一个新的媒体文件并插入到数据库中。注意,这里我假设你已经定义了 MediaFile 实体类,并且数据库中有对应的 media_file 表。如果没有,请根据实际情况进行修改。
相关问题
@Override public void addAdvert(AdvertDTO advertDto) { //MediaFile mediaFile = new MediaFile(); AdPosition adPosition = Optional.ofNullable(adPositionMapper.selectByPrimaryKey(advertDto.getPositionId())) .orElseThrow(() -> new RuntimeException("没有找到对应的广告位置")); Advert advert = Advert.builder() .name(advertDto.getName()) .position(adPosition) .media(advertDto.getMediaFile()) .startDate(advertDto.getStartDate()) .endDate(advertDto.getEndDate()) .link(advertDto.getLink()) .status(advertDto.getStatus()) .build(); AdvertMapper.insertSelective(advert); } @Override public void updateAdvert(Integer id, AdvertDTO advertDto) { AdPosition adPosition = Optional.ofNullable(adPositionMapper.selectByPrimaryKey(advertDto.getPositionId())) .orElseThrow(() -> new RuntimeException("Ad position not found")); Advert advert = Advert.builder() .name(advertDto.getName()) .position(adPosition) .media(advertDto.getMediaFile()) .startDate(advertDto.getStartDate()) .endDate(advertDto.getEndDate()) .link(advertDto.getLink()) .status(null != advertDto.getStatus() ? advertDto.getStatus() : 0) .build(); AdvertMapper.insert(advert); },如何优化代码
首先需要注意的是,在 updateAdvert 方法中,我们应该使用 updateByPrimaryKeySelective 方法而不是 insert 方法,因为我们只需要更新部分字段而不是全部字段。
另外,在两个方法中,我们可以使用 AdvertMapper 的自动生成代码中的 updateByExampleSelective 方法和 insertSelective 方法,这样可以减少手动编写 SQL 语句的工作量,也可以更简洁地实现相同的功能。
最后,我们可以将 Optional.ofNullable().orElseThrow() 的代码块提取出来作为一个方法,这样可以使代码更加简洁易读,也可以提高代码的复用性。
综上所述,下面是优化后的代码示例:
```
private AdPosition getAdPositionById(Integer positionId) {
return Optional.ofNullable(adPositionMapper.selectByPrimaryKey(positionId))
.orElseThrow(() -> new RuntimeException("Ad position not found"));
}
@Override
public void addAdvert(AdvertDTO advertDto) {
Advert advert = Advert.builder()
.name(advertDto.getName())
.position(getAdPositionById(advertDto.getPositionId()))
.media(advertDto.getMediaFile())
.startDate(advertDto.getStartDate())
.endDate(advertDto.getEndDate())
.link(advertDto.getLink())
.status(advertDto.getStatus())
.build();
advertMapper.insertSelective(advert);
}
@Override
public void updateAdvert(Integer id, AdvertDTO advertDto) {
Advert advert = Advert.builder()
.name(advertDto.getName())
.position(getAdPositionById(advertDto.getPositionId()))
.media(advertDto.getMediaFile())
.startDate(advertDto.getStartDate())
.endDate(advertDto.getEndDate())
.link(advertDto.getLink())
.status(null != advertDto.getStatus() ? advertDto.getStatus() : 0)
.build();
AdvertExample example = new AdvertExample();
example.createCriteria().andIdEqualTo(id);
advertMapper.updateByExampleSelective(advert, example);
}
```
@Data @AllArgsConstructor @NoArgsConstructor @Builder @Table(name = "advert") public class Advert { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @ApiModelProperty(value = "广告唯一id") private Integer id; @ApiModelProperty(value = "广告名称") @NotBlank(message = "广告名称不可为空", groups = {AddGroup.class, UpdateGroup.class}) private String name; @ManyToOne(fetch = FetchType.LAZY) @JoinColumn(name = "position_id") private AdPosition position; @ManyToOne(fetch = FetchType.LAZY) @JoinColumn(name = "media_id") private MediaFile media; } @Data @AllArgsConstructor @NoArgsConstructor @Builder @Table(name = "ad_position") public class AdPosition { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Integer id; @ApiModelProperty(value = "位置名称") @NotBlank(message = "位置名称不可为空", groups = {AddGroup.class}) private String name; @OneToMany(mappedBy = "AdPosition", cascade = CascadeType.ALL) private List<Advert> adverts = new ArrayList<>(); } @Data @AllArgsConstructor @NoArgsConstructor @Builder @Table(name = "media_file") public class MediaFile { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Integer id; @ApiModelProperty(value = "媒体文件名称") private String fileName; @ApiModelProperty(value = "媒体文件类型") private Integer fileType; @ApiModelProperty(value = "媒体文件URL地址") private String fileUrl; @OneToMany(mappedBy = "MediaFile", cascade = CascadeType.ALL) private List<Advert> adverts = new ArrayList<>(); } public interface MyBaseMapper<T> extends Mapper<T>, MySqlMapper<T>,MyInsertListMapper<T> {} public interface AdvertMapper extends MyBaseMapper<Advert> {} @Autowired private AdvertMapper advertMapper; AdPosition adPosition = Optional.ofNullable(adPositionMapper.selectByPrimaryKey(advertDto.getPositionId())) .orElseThrow(() -> new RuntimeException("Ad position not found")); // 创建一个新的Advert对象 Advert advert = Advert.builder() .name("广告名称") .position(adPosition) // 假设AdPosition表中id为1的记录是需要的位置 .media(new MediaFile(1)) // 假设MediaFile表中id为1的记录是需要的媒体文件 .build(); // 调用insertSelective方法插入数据 advertMapper.insertSelective(advert);,报错Field 'position_id' doesn't have a default value ; Field 'position_id' doesn't have a default value; nested exception is java.sql.SQLException: Field 'position_id' doesn't have a default value,为什么
这个错误是由于在Advert表中的position_id字段没有设置默认值且未被赋值,在执行插入操作时,MySQL会抛出该异常。解决方法是在Advert表中的position_id字段上加上默认值或者在插入操作中给该字段赋值。例如在Advert实体类中的position字段上加上@JoinColumn注解的nullable属性,将它设置为true,表示该字段可以为null。或者在插入操作中给position_id字段赋值,例如advert.setPosition(adPosition)。
阅读全文
相关推荐



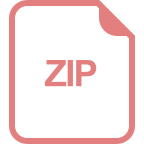




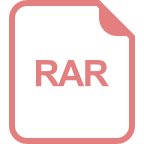







