python写一个小蜜蜂游戏
时间: 2023-07-07 12:39:01 浏览: 79
好的,下面是一个简单的Python小蜜蜂游戏:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口的宽和高
width = 600
height = 600
# 创建游戏窗口
screen = pygame.display.set_mode((width, height))
# 设置游戏窗口标题
pygame.display.set_caption("小蜜蜂游戏")
# 加载小蜜蜂图片
bee_img = pygame.image.load("bee.png")
# 设置小蜜蜂的初始位置和速度
bee_x = 250
bee_y = 500
bee_speed = 5
# 创建蜜蜂列表和速度列表
bees = []
bee_speeds = []
# 初始化蜜蜂列表和速度列表
for i in range(10):
bees.append(pygame.Rect(random.randint(0, width-50), random.randint(-500, 0), 50, 50))
bee_speeds.append(random.randint(1, 5))
# 加载背景音乐
pygame.mixer.music.load("bg_music.mp3")
pygame.mixer.music.set_volume(0.2)
pygame.mixer.music.play(-1)
# 创建字体对象
font = pygame.font.Font(None, 36)
# 初始化得分和游戏结束标志
score = 0
game_over = False
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
bee_x -= bee_speed
elif event.key == pygame.K_RIGHT:
bee_x += bee_speed
# 移动蜜蜂
for i in range(10):
bees[i].move_ip(0, bee_speeds[i])
if bees[i].bottom > height:
bees[i].top = random.randint(-500, 0)
bees[i].left = random.randint(0, width-50)
bee_speeds[i] = random.randint(1, 5)
score += 1
# 清除上一帧画面
screen.fill((255, 255, 255))
# 绘制小蜜蜂和蜜蜂
screen.blit(bee_img, (bee_x, bee_y))
for bee in bees:
pygame.draw.rect(screen, (255, 0, 0), bee)
# 绘制得分
score_text = font.render("得分:%d" % score, True, (0, 0, 0))
screen.blit(score_text, (10, 10))
# 判断游戏是否结束
for bee in bees:
if bee.colliderect(pygame.Rect(bee_x, bee_y, 50, 50)):
game_over = True
break
if game_over:
game_over_text = font.render("游戏结束!", True, (255, 0, 0))
screen.blit(game_over_text, (width//2 - 100, height//2 - 20))
# 更新屏幕显示
pygame.display.update()
```
在运行这个程序之前,你需要准备以下素材文件:
- bee.png:小蜜蜂的图片文件
- bg_music.mp3:游戏的背景音乐文件
将这两个文件放在程序同级目录下,然后运行程序即可开始游戏。在游戏中,你需要控制小蜜蜂躲避下落的蜜蜂,并尽可能地吃到更多的蜜蜂,得分越高,游戏难度也会相应提高。如果小蜜蜂被蜜蜂撞到,游戏就会结束。
相关推荐
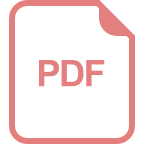





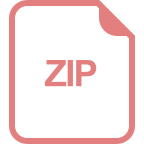
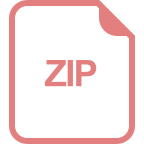
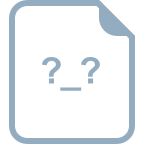
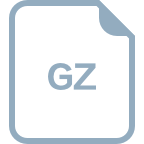