django 为上传的视频添加字幕
时间: 2024-05-09 21:16:16 浏览: 14
要为上传的视频添加字幕,可以使用 Django 中的第三方库 django-ffmpeg。以下是一个基本的示例:
首先,安装 django-ffmpeg:`pip install django-ffmpeg`
然后,在你的 Django 项目中的 settings.py 文件中添加以下内容:
```
FFMPEG_PATH = '/usr/bin/ffmpeg' # 设置 FFmpeg 的路径
FFPROBE_PATH = '/usr/bin/ffprobe' # 设置 FFprobe 的路径
```
接下来,创建一个新的 Django 视图来处理上传的视频:
```
from django.views.generic.edit import CreateView
from django.urls import reverse_lazy
from django_ffmpeg.views import VideoConvertView
from myapp.models import Video
class VideoCreateView(CreateView):
model = Video
fields = ['title', 'video_file', 'subtitle_file']
success_url = reverse_lazy('video_list')
def form_valid(self, form):
response = super().form_valid(form)
video = form.instance
# 使用 django-ffmpeg 将上传的视频转换为 mp4 格式
convert_view = VideoConvertView.as_view()
request = self.request
request.method = 'POST'
request.POST = {
'input': video.video_file.path,
'output': video.video_file.path.replace('.mov', '.mp4'),
'format': 'mp4',
}
response = convert_view(request)
# 如果成功转换为 mp4 格式,则添加字幕
if response.status_code == 200:
subtitle_file = form.cleaned_data.get('subtitle_file')
if subtitle_file:
command = [
FFMPEG_PATH,
'-i', video.video_file.path.replace('.mov', '.mp4'),
'-vf', f"subtitles='{subtitle_file.path}':force_style='Fontsize=24'",
'-c:a', 'copy',
video.video_file.path.replace('.mov', '_with_subtitles.mp4'),
]
subprocess.call(command)
return response
```
在这个示例中,我们首先使用 django-ffmpeg 将上传的视频转换为 mp4 格式。如果转换成功,则使用 FFmpeg 将字幕添加到视频中。
需要注意的是,添加字幕需要 FFmpeg 版本 4.0 或更高版本。
希望这个示例可以帮助你为上传的视频添加字幕。
相关推荐
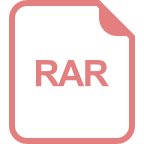
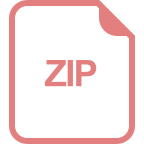
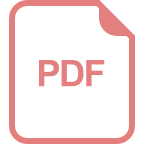














