java导出excel数据量大压缩包
时间: 2023-06-26 13:07:39 浏览: 114
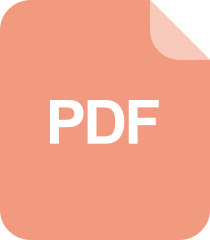
java导出大批量(百万以上)数据的excel文件
如果你需要处理大量数据并将其导出为Excel文件,可以使用Java的Apache POI库。而如果你想将导出的Excel文件进行压缩,可以使用Java的ZipOutputStream类来创建压缩包。
以下是一个示例代码,它使用Apache POI库将数据导出为Excel文件,并使用ZipOutputStream类将其压缩:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelZipExporter {
public static void main(String[] args) {
String[] headers = {"Name", "Age", "Email"};
Object[][] data = {{"John", 25, "john@example.com"}, {"Mary", 30, "mary@example.com"}};
try (ZipOutputStream zipOut = new ZipOutputStream(new FileOutputStream("data.zip"));
XSSFWorkbook workbook = new XSSFWorkbook()) {
Sheet sheet = workbook.createSheet("Data");
Row headerRow = sheet.createRow(0);
for (int i = 0; i < headers.length; i++) {
Cell cell = headerRow.createCell(i);
cell.setCellValue(headers[i]);
}
for (int rowIndex = 1; rowIndex <= data.length; rowIndex++) {
Row row = sheet.createRow(rowIndex);
for (int colIndex = 0; colIndex < data[rowIndex - 1].length; colIndex++) {
Cell cell = row.createCell(colIndex);
cell.setCellValue(data[rowIndex - 1][colIndex].toString());
}
}
// Write Excel file
workbook.write(new FileOutputStream("data.xlsx"));
// Add Excel file to ZIP archive
ZipEntry zipEntry = new ZipEntry("data.xlsx");
zipOut.putNextEntry(zipEntry);
byte[] buffer = new byte[1024];
int length;
try (FileInputStream fis = new FileInputStream("data.xlsx")) {
while ((length = fis.read(buffer)) > 0) {
zipOut.write(buffer, 0, length);
}
}
zipOut.closeEntry();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
该代码将数据导出为Excel文件,并将其添加到名为“data.xlsx”的ZIP文件中。你可以通过修改数据数组来添加更多数据并导出更大的Excel文件。
阅读全文
相关推荐
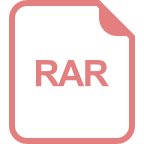
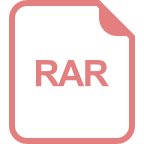














