用jsp+struts2框架代码实现以下功能:导出千万级数据量,要求每50000笔数据导入到excel表格直到导完为止,再讲得到的多个excel表格压缩成zip包,最后导出为一个压缩包
时间: 2023-08-02 08:07:17 浏览: 212
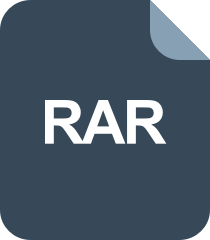
java导出30万数据量的excel(采用生成多个excel,最后打包zip)

以下是实现该功能的代码示例:
1. 编写Action类,实现数据导出功能
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
import javax.servlet.http.HttpServletResponse;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Font;
import org.apache.poi.ss.usermodel.HorizontalAlignment;
import org.apache.poi.ss.usermodel.IndexedColors;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.struts2.ServletActionContext;
public class ExportAction {
// 要导出的数据列表
private List<Data> dataList;
// 每次导出的数据量
private static final int BATCH_SIZE = 50000;
public String execute() throws Exception {
HttpServletResponse response = ServletActionContext.getResponse();
response.setContentType("application/zip");
response.setHeader("Content-Disposition", "attachment; filename=data.zip");
// 创建临时文件夹
File tempDir = new File(System.getProperty("java.io.tmpdir"), "data");
if (!tempDir.exists()) {
tempDir.mkdirs();
}
// 按照BATCH_SIZE拆分数据,每批数据导出到一个Excel文件中
int batchCount = (dataList.size() - 1) / BATCH_SIZE + 1;
List<File> excelFiles = new ArrayList<File>();
for (int i = 0; i < batchCount; i++) {
int startIndex = i * BATCH_SIZE;
int endIndex = Math.min(startIndex + BATCH_SIZE, dataList.size());
List<Data> batchData = dataList.subList(startIndex, endIndex);
File excelFile = new File(tempDir, "data-" + (i + 1) + ".xls");
exportToExcel(batchData, excelFile);
excelFiles.add(excelFile);
}
// 把多个Excel文件压缩成一个ZIP文件
File zipFile = new File(tempDir, "data.zip");
zipFiles(excelFiles, zipFile);
// 输出ZIP文件
OutputStream out = response.getOutputStream();
FileInputStream in = new FileInputStream(zipFile);
byte[] buffer = new byte[1024];
int len = 0;
while ((len = in.read(buffer)) != -1) {
out.write(buffer, 0, len);
}
in.close();
out.close();
// 删除临时文件夹及其内容
deleteDirectory(tempDir);
return null;
}
// 把数据导出到Excel文件中
private void exportToExcel(List<Data> data, File file) throws IOException {
Workbook wb = new HSSFWorkbook();
Sheet sheet = wb.createSheet("Data");
// 设置表头样式
CellStyle headerStyle = wb.createCellStyle();
Font headerFont = wb.createFont();
headerFont.setBold(true);
headerFont.setColor(IndexedColors.WHITE.getIndex());
headerStyle.setFillForegroundColor(IndexedColors.BLUE.getIndex());
headerStyle.setFillPattern(CellStyle.SOLID_FOREGROUND);
headerStyle.setAlignment(HorizontalAlignment.CENTER);
headerStyle.setFont(headerFont);
// 创建表头
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("ID");
headerRow.createCell(1).setCellValue("Name");
headerRow.createCell(2).setCellValue("Age");
headerRow.createCell(3).setCellValue("Address");
for (int i = 0; i < headerRow.getLastCellNum(); i++) {
headerRow.getCell(i).setCellStyle(headerStyle);
sheet.setColumnWidth(i, 20 * 256); // 设置列宽
}
// 设置内容样式
CellStyle contentStyle = wb.createCellStyle();
Font contentFont = wb.createFont();
contentFont.setColor(IndexedColors.BLACK.getIndex());
contentStyle.setFont(contentFont);
// 填充数据
for (int i = 0; i < data.size(); i++) {
Data d = data.get(i);
Row row = sheet.createRow(i + 1);
row.createCell(0).setCellValue(d.getId());
row.createCell(1).setCellValue(d.getName());
row.createCell(2).setCellValue(d.getAge());
row.createCell(3).setCellValue(d.getAddress());
for (int j = 0; j < row.getLastCellNum(); j++) {
row.getCell(j).setCellStyle(contentStyle);
}
}
// 输出Excel文件
FileOutputStream out = new FileOutputStream(file);
wb.write(out);
out.close();
}
// 把多个文件压缩成一个ZIP文件
private void zipFiles(List<File> files, File zipFile) throws IOException {
byte[] buffer = new byte[1024];
ZipOutputStream out = new ZipOutputStream(new FileOutputStream(zipFile));
for (File file : files) {
FileInputStream in = new FileInputStream(file);
out.putNextEntry(new ZipEntry(file.getName()));
int len = 0;
while ((len = in.read(buffer)) != -1) {
out.write(buffer, 0, len);
}
in.close();
}
out.close();
}
// 删除目录及其内容
private void deleteDirectory(File dir) {
if (dir.isDirectory()) {
for (File file : dir.listFiles()) {
deleteDirectory(file);
}
}
dir.delete();
}
public List<Data> getDataList() {
return dataList;
}
public void setDataList(List<Data> dataList) {
this.dataList = dataList;
}
}
```
2. JSP页面上添加导出按钮,并调用Action类的execute方法
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib uri="/struts-tags" prefix="s"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Export Data</title>
</head>
<body>
<h1>Export Data</h1>
<s:form action="export">
<s:submit value="Export"/>
</s:form>
</body>
</html>
```
在实际使用中,需要将数据列表传递给Action类,并在exportToExcel方法中根据实际数据结构填充Excel文件。此外,还需要在struts.xml中配置Action类的映射关系。
阅读全文
相关推荐
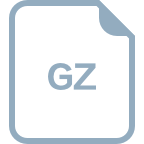

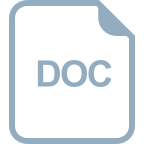
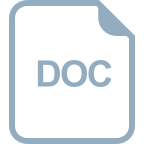
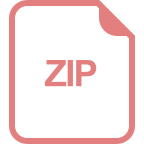
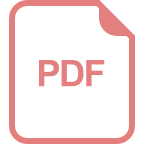
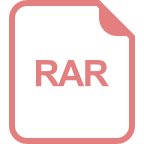
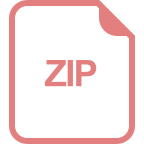
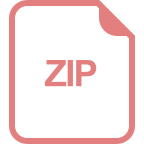
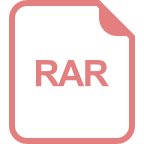
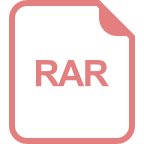
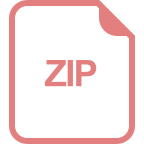
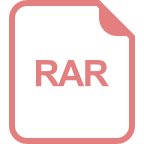
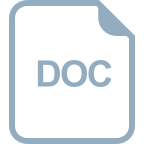
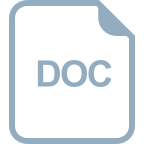
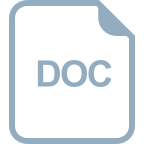
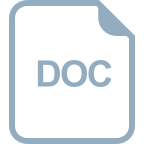