strus2如何实现导入导出带图片的数据到excel
时间: 2024-02-17 14:00:22 浏览: 79
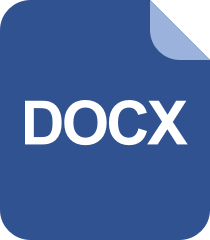
struts2实现excel导出
在 Struts2 中,可以使用 Apache POI 库来实现导入导出带图片的数据到 Excel。POI 提供了 HSSF 和 XSSF 两个 API 来操作 Excel 文件,其中 HSSF 主要用于处理 Excel 2003 及之前的版本,而 XSSF 则主要用于处理 Excel 2007 及之后的版本。
下面是一个简单的示例,演示了如何使用 POI 在 Struts2 中导出带图片的数据到 Excel:
1. 首先,需要添加 Apache POI 和 Apache FileUpload 依赖。可以在 `pom.xml` 文件中添加以下内容:
```
<dependencies>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
</dependencies>
```
2. 创建一个 `ExportAction` 类,该类用于处理导出请求。在 `execute()` 方法中,可以使用 POI 创建一个新的工作簿,并将数据和图片添加到工作簿中。最后,将工作簿写入响应输出流,以便用户可以下载 Excel 文件。
```
public class ExportAction extends ActionSupport {
private File upload; // 上传的文件
private String uploadContentType; // 上传文件的类型
private String uploadFileName; // 上传文件的名称
public String execute() throws Exception {
// 创建一个新的工作簿
Workbook workbook = new XSSFWorkbook();
// 创建一个新的工作表
Sheet sheet = workbook.createSheet("Sheet1");
// 创建表头行
Row headerRow = sheet.createRow(0);
// 创建表头单元格
Cell headerCell1 = headerRow.createCell(0);
headerCell1.setCellValue("ID");
Cell headerCell2 = headerRow.createCell(1);
headerCell2.setCellValue("名称");
Cell headerCell3 = headerRow.createCell(2);
headerCell3.setCellValue("图片");
// 读取上传的文件
InputStream input = new FileInputStream(upload);
// 使用 Apache FileUpload 解析上传的文件
DiskFileItemFactory factory = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(factory);
List<FileItem> items = upload.parseRequest(request);
// 遍历上传的文件项,将图片插入到工作表中
int rowIndex = 1;
for (FileItem item : items) {
if (!item.isFormField()) {
// 获取文件名和文件内容
String fileName = item.getName();
byte[] fileContent = item.get();
// 创建单元格,将图片插入到工作表中
Row row = sheet.createRow(rowIndex++);
Cell cell1 = row.createCell(0);
cell1.setCellValue(rowIndex - 1);
Cell cell2 = row.createCell(1);
cell2.setCellValue(fileName);
Cell cell3 = row.createCell(2);
// 将字节数组转换为图片并插入到单元格中
int pictureIndex = workbook.addPicture(fileContent, Workbook.PICTURE_TYPE_JPEG);
CreationHelper helper = workbook.getCreationHelper();
Drawing drawing = sheet.createDrawingPatriarch();
ClientAnchor anchor = helper.createClientAnchor();
anchor.setCol1(cell3.getColumnIndex());
anchor.setRow1(row.getRowNum());
Picture picture = drawing.createPicture(anchor, pictureIndex);
picture.resize(1, 1);
}
}
// 将工作簿写入响应输出流
response.setContentType("application/vnd.ms-excel");
response.setHeader("Content-Disposition", "attachment; filename=data.xlsx");
workbook.write(response.getOutputStream());
workbook.close();
return null;
}
// 省略 getter 和 setter 方法
}
```
3. 创建一个 JSP 页面,该页面包含一个表单,用户可以上传包含数据和图片的 Excel 文件。在表单中,需要将表单的 `enctype` 属性设置为 `multipart/form-data`,以便可以上传二进制文件。
```
<%@ taglib prefix="s" uri="/struts-tags" %>
<html>
<head>
<title>导入导出带图片的数据到 Excel</title>
</head>
<body>
<h1>导入导出带图片的数据到 Excel</h1>
<s:form action="export" method="post" enctype="multipart/form-data">
<s:file name="upload" label="上传 Excel 文件"/>
<s:submit value="导出"/>
</s:form>
</body>
</html>
```
以上就是在 Struts2 中实现导入导出带图片的数据到 Excel 的简单示例。请注意,此示例仅用于演示目的,实际应用中可能需要进行更多的错误处理和数据验证。
阅读全文
相关推荐
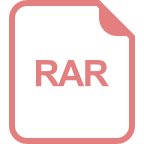
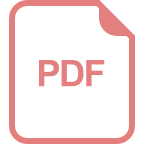
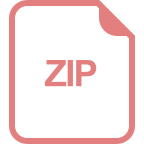
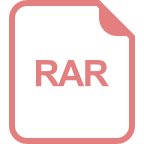
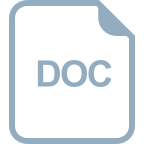
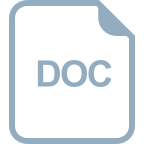
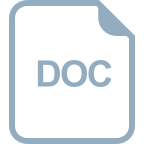
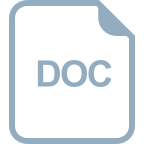
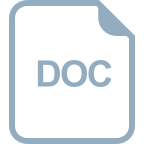
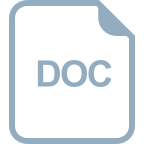
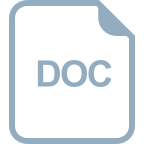
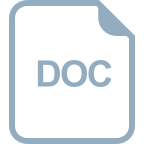
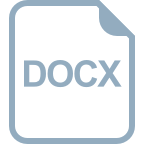
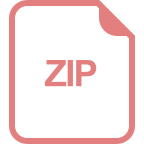
