设计一个低、中、高级的带界面的PC端的程序,低级可以计算十道10以内的数学加减法题,中级可以计算十道100以内的数学加减法题,高级可以计算十道1000以内的数学加减法题,计算每正确一次加一分,错误则不得分,计算结束时,分数为7分及以上则为闯关成功,7分一下则为闯关失败。
时间: 2024-02-24 11:56:45 浏览: 30
好的,以下是基本的设计思路:
1. 界面设计:可以使用GUI库(如PyQt、Tkinter)来设计带有交互式界面的小游戏。
2. 随机生成题目:根据用户所选的难度等级,程序可以随机生成对应难度的加减法题目。
3. 计分规则:每回答一个问题,程序会根据用户的回答情况(正确或错误)来判断是否加分。正确回答加1分,错误回答不得分。
4. 闯关机制:程序会根据用户的得分情况来判断是否通过本次闯关。当得分为7分或以上时,闯关成功,否则闯关失败。
5. 程序流程:根据用户所选的难度等级,程序会自动生成对应数量的数学题目,并在界面上呈现出来。用户需要在规定的时间内完成所有的题目,并得到相应的得分。
您可以参考以下代码实现:
```python
import random
import time
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLabel, QLineEdit, QVBoxLayout, QHBoxLayout, QRadioButton, QGroupBox, QMessageBox
class MathGame(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle('Math Game')
self.setGeometry(300, 300, 500, 300)
self.score = 0
self.timer = None
self.difficulty_group = QGroupBox('Difficulty Level')
self.difficulty_group_layout = QVBoxLayout()
self.difficulty_group.setLayout(self.difficulty_group_layout)
self.low_rbtn = QRadioButton('Low')
self.mid_rbtn = QRadioButton('Mid')
self.high_rbtn = QRadioButton('High')
self.difficulty_group_layout.addWidget(self.low_rbtn)
self.difficulty_group_layout.addWidget(self.mid_rbtn)
self.difficulty_group_layout.addWidget(self.high_rbtn)
self.low_rbtn.setChecked(True)
self.start_btn = QPushButton('Start')
self.start_btn.clicked.connect(self.start_game)
self.timer_lbl = QLabel('Time Left: 0')
self.score_lbl = QLabel('Score: 0')
self.question_lbl = QLabel('Question:')
self.answer_edit = QLineEdit()
self.submit_btn = QPushButton('Submit')
self.submit_btn.clicked.connect(self.submit_answer)
self.main_layout = QVBoxLayout()
self.main_layout.addWidget(self.difficulty_group)
self.main_layout.addWidget(self.start_btn)
self.main_layout.addWidget(self.timer_lbl)
self.main_layout.addWidget(self.score_lbl)
self.main_layout.addWidget(self.question_lbl)
self.main_layout.addWidget(self.answer_edit)
self.main_layout.addWidget(self.submit_btn)
self.setLayout(self.main_layout)
def start_game(self):
self.score = 0
self.score_lbl.setText(f'Score: {self.score}')
self.timer = 60
self.timer_lbl.setText(f'Time Left: {self.timer}')
self.start_btn.setEnabled(False)
difficulty_level = ''
if self.low_rbtn.isChecked():
difficulty_level = 'low'
elif self.mid_rbtn.isChecked():
difficulty_level = 'mid'
elif self.high_rbtn.isChecked():
difficulty_level = 'high'
self.question_list = self.generate_questions(difficulty_level)
self.current_question = 0
self.show_question()
self.timer_thread = TimerThread(self)
self.timer_thread.timer_signal.connect(self.update_timer)
self.timer_thread.start()
def show_question(self):
question = self.question_list[self.current_question]
self.question_lbl.setText(f'Question: {question}')
def submit_answer(self):
answer = self.answer_edit.text()
if answer == str(eval(self.question_list[self.current_question])):
self.score += 1
self.score_lbl.setText(f'Score: {self.score}')
QMessageBox.information(self, 'Correct', 'Your answer is correct!')
else:
QMessageBox.warning(self, 'Wrong', 'Your answer is wrong!')
self.current_question += 1
if self.current_question < len(self.question_list):
self.show_question()
else:
self.end_game()
def end_game(self):
self.timer_thread.terminate()
if self.score >= 7:
QMessageBox.information(self, 'Success', 'You pass the game!')
else:
QMessageBox.warning(self, 'Fail', 'You fail the game!')
self.start_btn.setEnabled(True)
def generate_questions(self, difficulty_level):
question_list = []
if difficulty_level == 'low':
for i in range(10):
a = random.randint(0, 10)
b = random.randint(0, 10)
operator = random.choice(['+', '-'])
question_list.append(f'{a} {operator} {b}')
elif difficulty_level == 'mid':
for i in range(10):
a = random.randint(0, 100)
b = random.randint(0, 100)
operator = random.choice(['+', '-'])
question_list.append(f'{a} {operator} {b}')
elif difficulty_level == 'high':
for i in range(10):
a = random.randint(0, 1000)
b = random.randint(0, 1000)
operator = random.choice(['+', '-'])
question_list.append(f'{a} {operator} {b}')
return question_list
def update_timer(self, time_left):
self.timer_lbl.setText(f'Time Left: {time_left}')
class TimerThread(QThread):
timer_signal = pyqtSignal(int)
def __init__(self, parent=None):
super().__init__(parent=parent)
self.time_left = 60
def run(self):
while self.time_left > 0:
self.time_left -= 1
self.timer_signal.emit(self.time_left)
time.sleep(1)
if __name__ == '__main__':
app = QApplication([])
math_game = MathGame()
math_game.show()
app.exec_()
```
这是一个基本的PC端数学小游戏,难度等级分为低、中、高三个等级,用户可以选择不同的难度等级来挑战不同难度的数学题目。程序会根据用户所选的难度等级来生成相应数量的数学题目,并在界面上呈现出来。用户需要在规定的时间内完成所有的题目,并得到相应的得分。程序会根据用户的得分情况来判断是否通过本次闯关。当得分为7分或以上时,闯关成功,否则闯关失败。
相关推荐
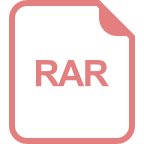














