编写一个程序动态分配一个浮点空间( double ),输入一个数到该空间中,计算以该数为半径的圆的面积并在屏幕上显示,最后释放该空间。请使用 new . delete 运算符.
时间: 2023-03-23 15:01:52 浏览: 116
好的,这是一个关于动态内存分配和计算圆面积的问题,我可以回答。
以下是示例程序:
```c++
#include <iostream>
int main()
{
double* radius = new double; // 分配浮点空间
std::cout << "请输入半径: ";
std::cin >> *radius; // 输入半径
double area = 3.1415926 * (*radius) * (*radius); // 计算面积
std::cout << "以" << *radius << "为半径的圆的面积为: " << area << std::endl;
delete radius; // 释放内存
return 0;
}
```
程序首先使用 `new` 运算符分配一个 `double` 类型的内存空间,用于存储输入的半径。接着从控制台读取用户输入的半径,并计算以该半径为半径的圆的面积。最后,使用 `delete` 运算符释放内存空间,避免内存泄漏。
注意:在实际编程中,应该对分配内存的结果进行检查,确保分配成功后再进行后续操作,避免因分配内存失败而导致程序崩溃。此外,为了提高程序的可读性和可维护性,应该尽可能使用变量和注释来解释代码的含义和目的。
相关问题
用c写一个输入圆形半径,计算圆形的面积和周长的程序
### 回答1:
您好,以下是用 C 语言编写的计算圆形面积和周长的程序:
#include <stdio.h>
#define PI 3.1415926535
int main()
{
double radius, area, perimeter;
printf("请输入圆形的半径:");
scanf("%lf", &radius);
area = PI * radius * radius;
perimeter = 2 * PI * radius;
printf("圆形的面积为:%lf\n", area);
printf("圆形的周长为:%lf\n", perimeter);
return ;
}
希望能够帮到您。
### 回答2:
要用C语言编写一个计算圆形面积和周长的程序,可以按照以下步骤进行:
步骤1:导入所需的头文件,包括stdio.h和math.h。其中math.h是为了使用数学函数pi。
步骤2:声明变量,包括圆形半径、圆形周长和圆形面积。数据类型可以选择浮点型或双精度型。
步骤3:使用printf函数输出提示用户输入圆形半径的信息。
步骤4:使用scanf函数接收用户输入的圆形半径并将其赋值给相应的变量。
步骤5:计算圆形周长和圆形面积,公式分别为:周长=2 * pi * 半径,面积=pi * 半径 * 半径。其中,pi为数学库中的常量。
步骤6:使用printf函数输出计算结果,包括圆形周长和圆形面积。
步骤7:结束程序。
以下是一个示例程序:
#include <stdio.h>
#include <math.h>
int main() {
float radius, circumference, area;
const float pi = 3.14159;
printf("请输入圆形的半径:");
scanf("%f", &radius);
circumference = 2 * pi * radius;
area = pi * radius * radius;
printf("圆形的周长为:%f\n", circumference);
printf("圆形的面积为:%f\n", area);
return 0;
}
上述的程序可以接收用户输入的圆形半径,并计算出圆形的周长和面积,并将结果输出给用户。
### 回答3:
下面是一个用C语言编写的程序,用于计算圆形的面积和周长:
```c
#include <stdio.h>
#define PI 3.1415926
int main() {
double radius, area, circumference;
printf("请输入圆的半径:");
scanf("%lf", &radius);
area = PI * radius * radius;
circumference = 2 * PI * radius;
printf("圆的面积为:%.2lf\n", area);
printf("圆的周长为:%.2lf\n", circumference);
return 0;
}
```
程序中使用了#include命令包含了stdio.h头文件,该头文件包含了输入输出的函数,比如printf和scanf。我们定义了一个常量PI来表示圆周率。在main函数中,首先定义了三个变量radius、area和circumference,用于存储输入的半径、计算的面积和周长。然后使用printf函数提示用户输入圆的半径,并使用scanf函数接收用户的输入。接下来,使用给定的计算公式分别计算出圆的面积和周长。最后,使用printf函数输出结果。输出时使用%.2lf来控制输出的小数位数为2位。
用户在运行程序时,会先提示用户输入圆的半径,用户输入后,程序会计算出圆的面积和周长,并将结果格式化输出到屏幕上。
1、实验名称:C++基础练习 2、实验内容: ① 内联画数与函数重载。 ② 动态内存分配与释放 3、实验目的: ① 熟悉C++程序基本的输入/输出操作。 ② 掌握内联函数定义、两数调用和两数原型的方法。 国 堂据 C++内存的动态分配与释放方法。 4、实验题目: ① 编写内联函数求解 2x²+4x+5的值,并用主函数调用该函数。(令x为int 和 double两类型数据,并利用函数重载分别实现)。(测试两个数值,5和 5.2) ② 编写一个程序动态分配一个浮点空间(double),输入一个数到该空间中,计算以该数为半径的圆的面积并在屏幕上显示,最后释放该空间。请使用 new、delete 运算符。
好的,我可以回答这个问题。
①编写内联函数求解2x²+4x+5的值,并用主函数调用该函数。(令x为int和double两类型数据,并利用函数重载分别实现)。(测试两个数值,5和5.2)
答案如下:
```c
#include <stdio.h>
inline int func(int x)
{
return 2*x*x + 4*x + 5;
}
inline double func(double x)
{
return 2*x*x + 4*x + 5;
}
int main()
{
int x1 = 5;
double x2 = 5.2;
printf("int: %d\n", func(x1));
printf("double: %f\n", func(x2));
return 0;
}
```
②编写一个程序动态分配一个浮点空间(double),输入一个数到该空间中,计算以该数为半径的圆的面积并在屏幕上显示,最后释放该空间。请使用new、delete运算符。
答案如下:
```c
#include <stdio.h>
int main()
{
double* p = new double;
printf("请输入半径:");
scanf("%lf", p);
double area = 3.14159 * (*p) * (*p);
printf("圆的面积为:%f\n", area);
delete p;
return 0;
}
```
相关推荐
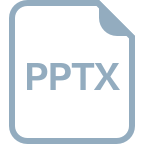
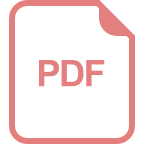
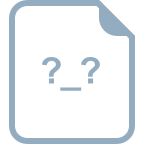













