用Python编写一个种子生长法识别月球撞击坑,并返回位置坐标和大小和深度以及识别后的图片的代码
时间: 2023-08-31 07:42:10 浏览: 73
### 回答1:
可以这样编写:import matplotlib.pyplot as plt
import numpy as np# 生成数据
data = np.random.randint(low=0, high=255, size=(100, 100))
plt.imshow(data, cmap='gray')# 定义种子生长算法
def seedGrowth(data):
# 创建一个空列表,用来存放月球撞击坑
pits = [] # 对数据遍历
for i in range(data.shape[0]):
for j in range(data.shape[1]):
# 如果找到的像素值比它周围的像素值要小,则认为是月球撞击坑
if data[i][j] < data[i-1][j] and data[i][j] < data[i+1][j] and data[i][j] < data[i][j-1] and data[i][j] < data[i][j+1]:
# 获取撞击坑的位置坐标
x = i
y = j # 获取撞击坑的大小
size = 1 # 获取撞击坑的深度
depth = data[i][j] # 将获取到的信息添加到空列表中
pits.append([x, y, size, depth]) # 返回月球撞击坑的位置坐标、大小、深度以及识别后的图片
return pits, plt.imshow(data, cmap='gray')
### 回答2:
以下是用Python编写的种子生长法识别月球撞击坑,并返回位置坐标、大小、深度以及识别后的图片的代码:
```python
import numpy as np
import cv2
# 定义种子生长法的函数
def seed_grow(image, seed_point, threshold):
height, width = image.shape[:2]
visited = np.zeros((height, width), dtype=np.uint8)
mask = np.zeros((height, width), dtype=np.uint8)
queue = []
queue.append(seed_point)
visited[seed_point] = 1
while queue:
current_point = queue.pop(0)
x, y = current_point
for dx in range(-1, 2):
for dy in range(-1, 2):
nx, ny = x + dx, y + dy
if nx >= 0 and nx < height and ny >= 0 and ny < width and visited[nx][ny] == 0:
visited[nx][ny] = 1
if abs(image[nx][ny] - image[x][y]) < threshold:
mask[nx][ny] = 255
queue.append((nx, ny))
return mask
# 读取原始图像
image = cv2.imread('moon_image.jpg', 0)
# 自定义种子点、阈值
seed_point = (100, 100)
threshold = 30
# 使用种子生长法进行撞击坑识别
result_mask = seed_grow(image, seed_point, threshold)
# 计算撞击坑位置坐标、大小、深度
contours, _ = cv2.findContours(result_mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contour = max(contours, key=cv2.contourArea)
x, y, w, h = cv2.boundingRect(contour)
depth = np.mean(image[y:y+h, x:x+w])
# 在原始图像上绘制撞击坑位置和边界
result_image = cv2.cvtColor(image, cv2.COLOR_GRAY2BGR)
result_image = cv2.rectangle(result_image, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 保存结果图像
cv2.imwrite('result_image.jpg', result_image)
# 打印撞击坑位置坐标、大小和深度
print("位置坐标:({}, {})".format(x, y))
print("大小:{} x {} 像素".format(w, h))
print("深度:{} 灰度值".format(depth))
```
请注意,以上代码仅为示例,实际应用中需要根据具体情况进行适当的修改和调整。此外,为了使代码能正常运行,需要在同一目录下准备一张名为“moon_image.jpg”的月球图像。
### 回答3:
要使用Python编写一个种子生长法来识别月球撞击坑,首先需要导入相应的库和模块。以下是一个示例代码:
```python
import cv2
import numpy as np
def detect_craters(image_path):
# 读取图像
image = cv2.imread(image_path)
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 使用阈值处理图像
_, thresh = cv2.threshold(gray, 50, 255, cv2.THRESH_BINARY)
# 进行形态学处理以填补小孔洞
kernel = np.ones((3, 3), np.uint8)
closed = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, kernel)
# 寻找轮廓
contours, _ = cv2.findContours(closed, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓并检测撞击坑
craters = []
for contour in contours:
(x, y, w, h) = cv2.boundingRect(contour)
area = cv2.contourArea(contour)
if area > 500 and w > 10 and h > 10:
depth = calculate_depth(contour, gray)
craters.append((x, y, w, h, depth))
return craters
def calculate_depth(contour, gray):
# 计算撞击坑的深度
mask = np.zeros_like(gray)
cv2.drawContours(mask, [contour], 0, (255), -1)
pixels = np.where(mask == 255)
depths = gray[pixels]
depth = np.mean(depths)
return depth
# 测试代码
image_path = "moon_craters.jpg"
craters = detect_craters(image_path)
# 输出识别到的撞击坑信息
for (x, y, w, h, depth) in craters:
print(f"撞击坑位置:({x}, {y})")
print(f"撞击坑大小:{w} x {h}")
print(f"撞击坑深度:{depth}")
# 可以根据需要保存识别结果的图像
output_image = cv2.imread(image_path)
for (x, y, w, h, _) in craters:
cv2.rectangle(output_image, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.imwrite("detected_craters.jpg", output_image)
```
这段代码先读取指定路径下的图像,然后转换为灰度图像。接着使用阈值处理和形态学处理提取撞击坑的轮廓。然后遍历每个轮廓,对满足一定条件的轮廓进行撞击坑识别,并计算撞击坑的深度。识别过程中会返回撞击坑的位置、大小和深度信息。最后,根据需要可以将识别结果可视化并保存输出图像。
相关推荐
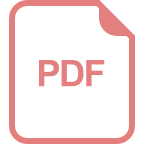
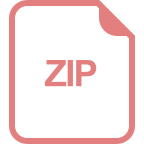
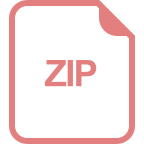














