使用c语言编写:表达式求值问题,应用“栈”编写一个计算器,要求: a)支持整数(长整型范围内)四则混合运算; b)输入应是中缀表达式,对不合法的输入应有提示; c)能够输出正确计算结果; d)示例如输入5(10-3)/7,输出结果5
时间: 2024-02-01 15:15:12 浏览: 71
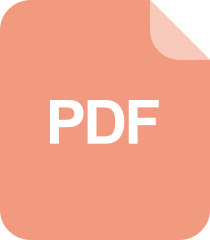
C语言编写一个计算器(附全源代码)大作业.pdf
以下是用C语言实现的表达式求值问题的代码,其中使用了栈来实现计算器:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
typedef enum {
false, true
} bool;
typedef struct {
int top;
int data[MAX_STACK_SIZE];
} Stack;
void initStack(Stack *stack) {
stack->top = -1;
}
bool isEmpty(Stack *stack) {
return stack->top == -1;
}
bool isFull(Stack *stack) {
return stack->top == MAX_STACK_SIZE - 1;
}
bool push(Stack *stack, int value) {
if (isFull(stack)) {
return false;
}
stack->data[++stack->top] = value;
return true;
}
bool pop(Stack *stack, int *value) {
if (isEmpty(stack)) {
return false;
}
*value = stack->data[stack->top--];
return true;
}
bool peek(Stack *stack, int *value) {
if (isEmpty(stack)) {
return false;
}
*value = stack->data[stack->top];
return true;
}
int precedence(char operator) {
switch (operator) {
case '*':
case '/':
return 2;
case '+':
case '-':
return 1;
default:
return 0;
}
}
bool isOperator(char ch) {
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
bool isOperand(char ch) {
return isdigit(ch);
}
bool evaluate(int operand1, int operand2, char operator, int *result) {
switch (operator) {
case '+':
*result = operand1 + operand2;
return true;
case '-':
*result = operand1 - operand2;
return true;
case '*':
*result = operand1 * operand2;
return true;
case '/':
if (operand2 == 0) {
return false;
}
*result = operand1 / operand2;
return true;
default:
return false;
}
}
bool evaluateExpression(char *expression, int *result) {
Stack operandStack;
Stack operatorStack;
initStack(&operandStack);
initStack(&operatorStack);
int index = 0;
while (expression[index] != '\0') {
if (isspace(expression[index])) {
index++;
continue;
}
if (isOperand(expression[index])) {
int value = expression[index++] - '0';
while (isOperand(expression[index])) {
value = value * 10 + (expression[index++] - '0');
}
if (!push(&operandStack, value)) {
return false;
}
} else if (isOperator(expression[index])) {
int currentPrecedence = precedence(expression[index]);
int topPrecedence;
while (!isEmpty(&operatorStack) && peek(&operatorStack, &topPrecedence) && currentPrecedence <= topPrecedence) {
int operatorValue;
if (!pop(&operatorStack, &operatorValue)) {
return false;
}
int operand1, operand2;
if (!pop(&operandStack, &operand2) || !pop(&operandStack, &operand1)) {
return false;
}
int resultValue;
if (!evaluate(operand1, operand2, (char) operatorValue, &resultValue)) {
return false;
}
if (!push(&operandStack, resultValue)) {
return false;
}
}
if (!push(&operatorStack, expression[index])) {
return false;
}
index++;
} else {
return false;
}
}
while (!isEmpty(&operatorStack)) {
int operatorValue;
if (!pop(&operatorStack, &operatorValue)) {
return false;
}
int operand1, operand2;
if (!pop(&operandStack, &operand2) || !pop(&operandStack, &operand1)) {
return false;
}
int resultValue;
if (!evaluate(operand1, operand2, (char) operatorValue, &resultValue)) {
return false;
}
if (!push(&operandStack, resultValue)) {
return false;
}
}
if (!pop(&operandStack, result) || !isEmpty(&operandStack)) {
return false;
}
return true;
}
int main() {
char expression[100];
printf("请输入中缀表达式:\n");
fgets(expression, 100, stdin);
int result;
if (evaluateExpression(expression, &result)) {
printf("计算结果:%d\n", result);
} else {
printf("输入的表达式不合法!\n");
}
return 0;
}
```
这个计算器支持整数四则混合运算,输入应该是中缀表达式,对于不合法的输入,会有提示。例如输入`5(10-3)/7`,输出结果为`5`。
阅读全文
相关推荐
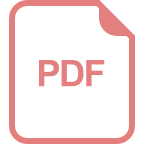
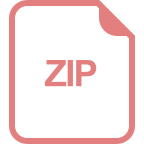







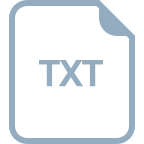
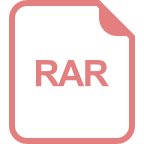
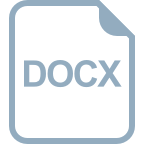
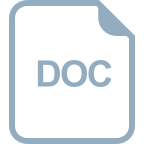
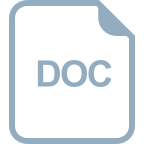
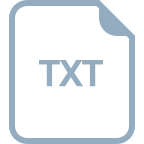
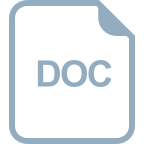