python开发数据库管理界面MySQL
时间: 2023-09-09 12:07:14 浏览: 61
Python有很多库可以用来开发数据库管理界面,其中最流行的是Tkinter和PyQt。以下是一个使用PyQt库开发MySQL数据库管理界面的示例代码:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QTableWidget, QTableWidgetItem, QVBoxLayout
import mysql.connector
class App(QWidget):
def __init__(self):
super().__init__()
self.title = 'MySQL Database Management'
self.left = 0
self.top = 0
self.width = 640
self.height = 480
self.initUI()
def initUI(self):
self.setWindowTitle(self.title)
self.setGeometry(self.left, self.top, self.width, self.height)
# Connect to the MySQL database
self.cnx = mysql.connector.connect(user='your_username', password='your_password',
host='your_host', database='your_database')
self.cursor = self.cnx.cursor()
# Create a table widget to display the data
self.tableWidget = QTableWidget()
self.tableWidget.setColumnCount(3)
self.tableWidget.setHorizontalHeaderLabels(['ID', 'Name', 'Age'])
# Populate the table with data from the database
self.populateTable()
# Add the table widget to a vertical layout and set the layout for the window
layout = QVBoxLayout()
layout.addWidget(self.tableWidget)
self.setLayout(layout)
# Show the window
self.show()
def populateTable(self):
self.cursor.execute('SELECT * FROM your_table')
rows = self.cursor.fetchall()
self.tableWidget.setRowCount(len(rows))
for i, row in enumerate(rows):
for j, col in enumerate(row):
self.tableWidget.setItem(i, j, QTableWidgetItem(str(col)))
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = App()
sys.exit(app.exec_())
```
在这个示例中,我们使用PyQt的QTableWidget组件来显示MySQL数据库中的数据。我们首先需要连接到数据库,然后从表中检索数据并将其填充到表格中。最后,我们将表格添加到一个垂直布局中,并将该布局设置为窗口的布局。运行该代码后,你将看到一个显示MySQL数据库中数据的窗口。
相关推荐
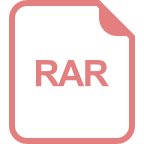
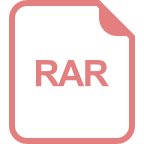
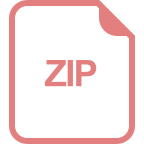
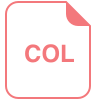
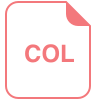
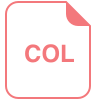
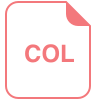
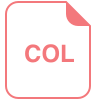









