C# json转成属性格式的xml
时间: 2023-07-02 18:22:28 浏览: 76
可以使用System.Xml.Serialization命名空间中的XmlSerializer类将JSON转换为XML。以下是一个示例代码:
```csharp
using System.Xml.Serialization;
using Newtonsoft.Json.Linq;
// 将JSON字符串转换为JObject对象
string jsonStr = "{'name': 'John', 'age': 30}";
JObject jsonObj = JObject.Parse(jsonStr);
// 将JObject对象转换为XML字符串
XmlSerializer serializer = new XmlSerializer(typeof(JObject));
using (StringWriter writer = new StringWriter())
{
serializer.Serialize(writer, jsonObj);
string xmlStr = writer.ToString();
Console.WriteLine(xmlStr);
}
```
注意:上面的代码需要引用Newtonsoft.Json和System.Xml.Serialization命名空间。
相关推荐
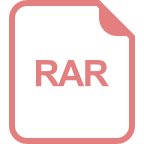
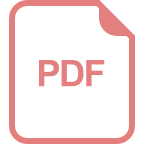
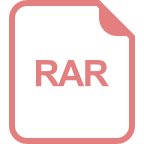
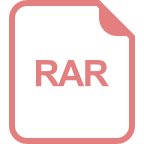
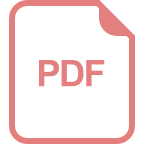
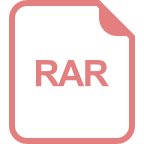
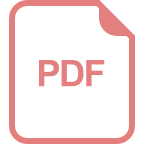
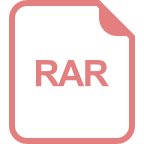
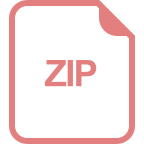
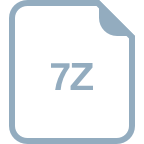
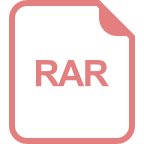
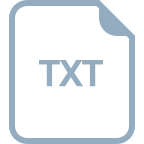
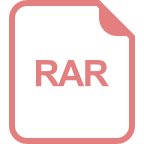
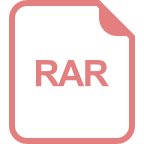
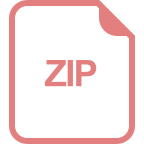
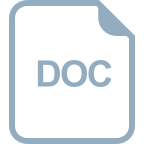