python爬取淘宝商品做数据分析的代码
时间: 2023-08-07 09:08:28 浏览: 57
以下是一个Python爬取淘宝商品并进行数据分析的代码示例,供您参考:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
import numpy as np
# 设置请求头
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 爬取淘宝商品页面
def get_taobao_products(keyword):
url = 'https://s.taobao.com/search?q=' + keyword
try:
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.text
except requests.exceptions.RequestException:
return None
# 解析HTML响应,提取商品信息
def parse_taobao_products(html):
soup = BeautifulSoup(html, 'html.parser')
products = []
for item in soup.find_all('div', class_='item'):
product = {
'name': item.find('a', class_='J_ClickStat')['title'],
'price': float(item.find('div', class_='price g_price g_price-highlight').text.strip()[1:]),
'sales': int(item.find('div', class_='deal-cnt').text.strip()[:-3]),
'shop': item.find('a', class_='shopname J_MouseEneterLeave J_ShopInfo').text.strip(),
'location': item.find('div', class_='location').text.strip()
}
products.append(product)
return products
# 将提取的数据存储到CSV文件中
def save_to_csv(products, file_path):
df = pd.DataFrame(products)
df.to_csv(file_path, index=False)
# 数据分析
def data_analysis(file_path):
df = pd.read_csv(file_path)
print('平均价格:', np.mean(df['price']))
print('销量排名:\n', df.sort_values(by='sales', ascending=False)['name'])
if __name__ == '__main__':
keyword = '手机'
html = get_taobao_products(keyword)
if html:
products = parse_taobao_products(html)
save_to_csv(products, 'products.csv')
data_analysis('products.csv')
else:
print('无法获取淘宝商品页面')
```
在运行代码之前,需要安装requests、BeautifulSoup、pandas和numpy等Python库。
在代码中,我们首先定义了一个函数`get_taobao_products`来爬取淘宝商品页面。然后,我们定义了一个函数`parse_taobao_products`来解析HTML响应,提取商品信息。接着,我们使用Pandas库将提取的数据存储到CSV文件中。最后,我们定义了一个函数`data_analysis`来进行数据分析,例如计算平均价格、销量排名等等。
在主函数中,我们设置了关键词为“手机”,并调用以上函数来爬取淘宝商品、进行数据分析。
相关推荐
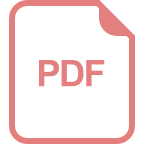
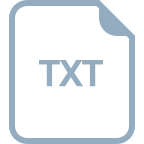
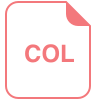
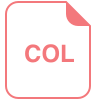
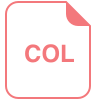
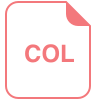
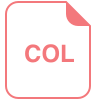









