python爬取百度网盘资源
时间: 2023-09-24 20:07:48 浏览: 56
要使用 Python 爬取百度网盘资源,可以使用第三方库 `requests` 和 `beautifulsoup4`。
首先,需要登录百度网盘获取到 cookies。可以使用 `requests.session()` 创建一个 session 对象,然后通过登录表单提交的方式获取到 cookies。
接下来,可以使用 `requests` 库发起 GET 请求,获取到网盘页面的 HTML。然后,使用 `beautifulsoup4` 库对 HTML 进行解析,提取需要的资源链接信息。
最后,可以使用 `requests` 库发起下载请求,下载资源文件。
以下是一个简单的代码示例:
```python
import requests
from bs4 import BeautifulSoup
# 登录百度网盘获取 cookies
session = requests.session()
login_url = 'https://passport.baidu.com/v2/api/?login'
data = {
'username': 'your_username',
'password': 'your_password'
}
session.post(login_url, data=data)
# 发起 GET 请求获取网盘页面 HTML
url = 'https://pan.baidu.com/s/your_share_link'
html = session.get(url).text
# 使用 BeautifulSoup 解析 HTML
soup = BeautifulSoup(html, 'html.parser')
links = soup.find_all('a')
# 获取资源链接并下载
for link in links:
href = link.get('href')
if href.startswith('https://pan.baidu.com/'):
download_url = href.replace('https://pan.baidu.com/', 'https://pan.baidu.com/api/sharedownload?')
data = {
'sign': soup.find('input', {'name': 'sign'}).get('value'),
'timestamp': soup.find('input', {'name': 'timestamp'}).get('value'),
'bdstoken': soup.find('input', {'name': 'bdstoken'}).get('value'),
'channel': 'chunlei',
'clienttype': '0',
'web': '1',
'app_id': '250528',
'encrypt': '0',
'product': 'share',
'uk': soup.find('input', {'name': 'uk'}).get('value'),
'shareid': soup.find('input', {'name': 'shareid'}).get('value'),
'filename': link.text,
'path': href.split('?')[0].split('/')[-1]
}
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = session.post(download_url, data=data, headers=headers)
with open(link.text, 'wb') as f:
f.write(response.content)
```
相关推荐
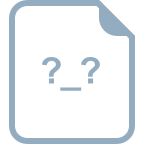















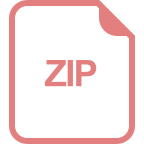