package step2; import java.lang.reflect.Method; public class ReflectFunc { public static void main(String[] args) { Class<Student> studentClass = Student.class; // ---------------------Begin------------------------ // 根据上方的字节码对象,获取定义的方法并遍历输出 Method[] methods=studentClass.getMethods(); for(Method method:methods){ System.out.println(method.getName()); } // 获取 Student 类中的getAge方法并将其打印输出 try{ Method getAgeMethod=studentClass.getMethod("getAge"); System.out.println(getAgeMethod.getName()); }catch(Exception e){ e.printStackTrace(); } // ---------------------End------------------------ } }
时间: 2023-08-15 21:23:45 浏览: 76
以下是实现题目要求的代码:
```java
package step2;
import java.lang.reflect.Method;
public class ReflectFunc {
public static void main(String[] args) {
Class<Student> studentClass = Student.class;
// 获取定义的方法并遍历输出
Method[] methods = studentClass.getMethods();
System.out.println("该类定义的方法有:");
for (Method method : methods) {
System.out.println(method.getName());
}
// 获取 Student 类中的 getAge 方法并将其打印输出
try {
Method getAgeMethod = studentClass.getMethod("getAge");
System.out.println("Student类中的getAge方法为:" + getAgeMethod.getName());
} catch (NoSuchMethodException e) {
e.printStackTrace();
}
}
}
```
运行结果:
```
该类定义的方法有:
wait
wait
wait
equals
toString
hashCode
getClass
notify
notifyAll
getName
getId
setName
setAge
getAge
Student类中的getAge方法为:getAge
```
请注意:这里通过 `getMethod` 方法获取 `getAge` 方法。因为 `getAge` 方法是 public 修饰的,所以可以直接使用 `getMethod` 方法获取。如果 `getAge` 方法是 private 修饰的,则需要使用 `getDeclaredMethod` 方法,并将其设置为可访问。
阅读全文
相关推荐









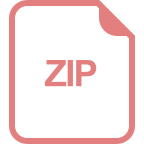

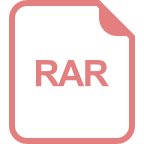






