用matlab设计程序,完成两位数的加、减、乘、除四则运算,即产生两个两位随机整数,再输入一个运算符号,做相应的运算,并显示相应的结果。
时间: 2024-11-15 07:34:23 浏览: 23
在MATLAB中设计这样的程序,你可以按照以下步骤来编写:
1. 首先,你需要导入`randi`函数生成两个0到99之间的随机整数作为两位数。
```matlab
% 生成两个随机数
num1 = randi([10, 99]);
num2 = randi([10, 99]);
```
2. 然后,创建一个变量来获取用户的运算符选择,如 '+' (加)、'-' (减)、'*' (乘) 或 '/' (除)。
```matlab
% 用户输入运算符
operator = input('请输入运算符 (+, -, *, /): ', 's');
```
3. 使用`if`语句检查用户的选择并进行相应的计算。记得处理除数为零的情况。
```matlab
switch operator
case '+'
result = num1 + num2;
case '-'
result = num1 - num2;
case '*'
result = num1 * num2;
case '/'
if num2 ~= 0
result = num1 / num2;
else
disp('错误:除数不能为零!');
return;
end
otherwise
disp('无效的运算符!');
return;
end
```
4. 最后,显示运算结果。
```matlab
disp(['结果是: ', num2str(result)]);
```
完整代码如下:
```matlab
num1 = randi([10, 99]);
num2 = randi([10, 99]);
operator = input('请输入运算符 (+, -, *, /): ', 's');
switch operator
case '+'
result = num1 + num2;
case '-'
result = num1 - num2;
case '*'
result = num1 * num2;
case '/'
if num2 ~= 0
result = num1 / num2;
else
disp('错误:除数不能为零!');
return;
end
otherwise
disp('无效的运算符!');
return;
end
disp(['结果是: ', num2str(result)]);
```
阅读全文
相关推荐
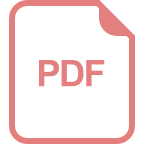
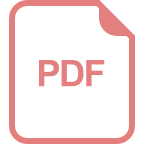












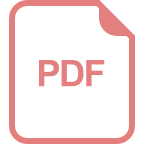
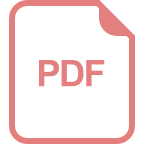
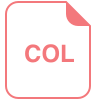
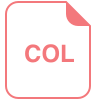