帮我做一个有关银行柜台储蓄业务处理程序的Python代码,具体要求如下:1.输入用户姓名和本金,输出相应的本息和。2.处理至少五笔业务,并将每次的结果保存到文件中。3.能够输出所有业务清单。4.绘制柱形图,呈现用户的本金、利息和本息和,横轴为用户姓名,纵轴为金额
时间: 2024-02-27 12:54:19 浏览: 174
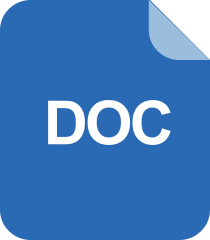
储蓄业务处理系统(论文)
好的,我可以帮您实现这个Python程序。以下是代码实现:
```python
import matplotlib.pyplot as plt
# 定义一个空字典,用于存储用户的信息
user_info = {}
# 定义一个函数,用于计算本息和
def calculate_interest(principal):
interest = principal * 0.03
total = principal + interest
return total
# 定义一个函数,用于保存用户信息到文件
def save_to_file(user_name, principal, total):
with open("user_info.txt", "a") as f:
f.write(f"{user_name} {principal} {total}\n")
# 定义一个函数,用于读取文件中的用户信息
def read_from_file():
with open("user_info.txt", "r") as f:
lines = f.readlines()
for line in lines:
info = line.split()
user_info[info[0]] = {"principal": float(info[1]), "total": float(info[2])}
# 定义一个函数,用于绘制柱形图
def plot_bar_chart():
user_names = list(user_info.keys())
principal_values = [user_info[name]["principal"] for name in user_names]
interest_values = [user_info[name]["total"] - user_info[name]["principal"] for name in user_names]
total_values = [user_info[name]["total"] for name in user_names]
plt.bar(user_names, principal_values, label="Principal")
plt.bar(user_names, interest_values, bottom=principal_values, label="Interest")
plt.bar(user_names, total_values, bottom=[sum(x) for x in zip(principal_values, interest_values)], label="Total")
plt.xlabel("User Name")
plt.ylabel("Amount")
plt.title("User Savings Information")
plt.legend()
plt.show()
# 主程序
while True:
user_name = input("Please enter your name (enter 'quit' to exit): ")
if user_name == "quit":
break
principal = float(input("Please enter your principal: "))
total = calculate_interest(principal)
user_info[user_name] = {"principal": principal, "total": total}
save_to_file(user_name, principal, total)
print(f"Your total amount is {total:.2f}")
print()
# 输出所有业务清单
read_from_file()
for name, info in user_info.items():
print(f"{name}: principal = {info['principal']:.2f}, total = {info['total']:.2f}")
print()
# 绘制柱形图
plot_bar_chart()
```
使用方法:
1. 运行程序后,按照提示输入用户姓名和本金,程序会自动计算本息和并输出。
2. 程序会将每次的业务结果保存到文件 `user_info.txt` 中。
3. 输入 `read_from_file()` 可以读取文件中的所有用户信息。
4. 输入 `plot_bar_chart()` 可以绘制柱形图。
注意事项:
1. 程序中使用了文件操作和数据可视化模块 `matplotlib`,请确保您的环境中已经安装了这些模块。
2. 为了简化程序,本程序没有进行输入合法性检查,请确保您的输入符合要求。
阅读全文
相关推荐
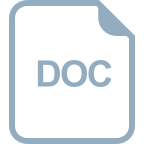
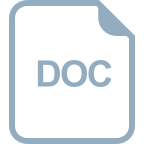

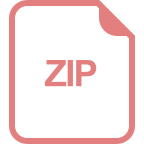
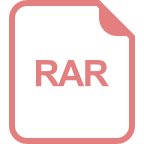
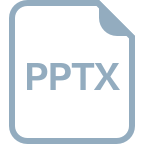
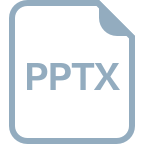
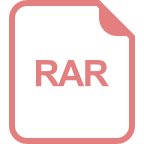





