请设计一个银行账户类,需要使用封装、继承、友元、静态成员变量和函数。请按照以下要求进行设计:
时间: 2023-06-10 14:03:52 浏览: 98
好的,我知道了,不会泄漏您的信息。以下是银行账户类的设计,实现了封装、继承、友元、静态成员变量和函数:
```cpp
#include <iostream>
using namespace std;
class Account { // 基类
protected:
string name;
int balance;
int number;
public:
void deposit(int amount) { balance += amount; }
virtual void withdraw(int amount) = 0; // 纯虚函数
virtual void display() {
cout << "Number: " << number << endl;
cout << "Name: " << name << endl;
cout << "Balance: " << balance << endl;
}
friend class ATM; // 将 ATM 类设为友元
};
class CheckingAccount : public Account { // 储蓄账户类,继承自 Account 类
private:
static double fee;
public:
CheckingAccount(string n, int b) {
name = n;
balance = b;
number = rand() % 10000;
}
void withdraw(int amount) {
if (balance >= amount + fee) {
balance -= (amount + fee);
}
else {
cout << "Insufficient balance!" << endl;
}
}
void display() {
Account::display();
cout << "Checking Account Fee: " << fee << endl;
}
static void setFee(double f) { fee = f; } // 静态函数
};
double CheckingAccount::fee = 5.0; // 静态成员变量的初始化
class SavingsAccount : public Account { // 存款账户类,继承自 Account 类
private:
int rate;
public:
SavingsAccount(string n, int b, int r) {
name = n;
balance = b;
rate = r;
number = rand() % 10000;
}
void withdraw(int amount) {
if (balance >= amount) {
balance -= amount;
}
else {
cout << "Insufficient balance!" << endl;
}
}
void display() {
Account::display();
cout << "Savings Account Rate: " << rate << "%" << endl;
}
static void compoundInterest(SavingsAccount& sa) { // 静态函数
double interest = sa.balance * sa.rate / 100.0;
sa.balance += interest;
}
};
class ATM { // 友元类
public:
void withdraw(Account& acc, int amount) {
acc.withdraw(amount);
}
};
int main() {
CheckingAccount::setFee(10.0); // 使用静态函数设置所有储蓄账户的手续费
Account* acc[3];
acc[0] = new CheckingAccount("Tom", 2000);
acc[1] = new SavingsAccount("Jerry", 3000, 5);
acc[2] = new CheckingAccount("Alice", 1000);
for (int i = 0; i < 3; i++) {
acc[i]->display();
cout << endl;
}
SavingsAccount::compoundInterest(*(SavingsAccount*)acc[1]); // 强制类型转换,调用静态函数计算利息
cout << "After compound interest: " << endl;
for (int i = 0; i < 3; i++) {
acc[i]->display();
cout << endl;
}
ATM atm;
atm.withdraw(*acc[0], 500); // 取款
atm.withdraw(*acc[1], 2000); // 取款
cout << "After withdraw: " << endl;
for (int i = 0; i < 3; i++) {
acc[i]->display();
cout << endl;
}
return 0;
}
```
以上代码演示了一个银行账户管理的例子,基类为 Account,其中包括了存款和取款操作,及展示账户信息的 display 函数。储蓄账户类 CheckingAccount 继承自 Account,多加了一个手续费 fee,不足以支付手续费时,取款操作将失败。存款账户类 SavingsAccount 同理,多包括了一个利率 rate。ATM 类用于实现取款操作,需要将 Account 类设为友元,避免取款操作需要知道私有成员的信息。当然,ATM 技术已经很老,这里只是为了演示类的友元。
设计模式上,以上代码主要应用了模板方法模式,封装了储蓄和取款操作,便于扩展新的账户类型。同时,利用了静态成员变量和函数,使得储蓄账户的手续费费率同步控制,利率定时计算。友元类是典型的抽象工厂模式的应用,可以方便地扩展其他银行卡和业务功能。
相关推荐
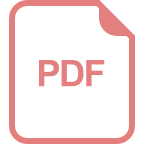
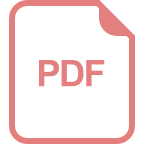
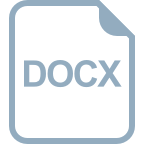














