pathon 用正则表达式来爬取评论
时间: 2023-12-25 21:45:44 浏览: 102
以下是使用 Python 的 re 模块来爬取评论的示例代码:
```python
import re
import requests
# 评论页面的 URL
url = 'https://example.com/comments'
# 发起 GET 请求,并获取响应内容
response = requests.get(url)
html = response.text
# 使用正则表达式匹配评论
pattern = r'<div class="comment">(.*?)</div>'
matches = re.findall(pattern, html, re.DOTALL)
# 输出所有匹配的评论
for match in matches:
print(match)
```
上述代码中,我们首先使用 requests 库来发起 GET 请求,并获取评论页面的 HTML 内容。然后,使用正则表达式来匹配评论。在这个例子中,我们假设评论是被包含在一个具有 "comment" 类的 div 元素中的。因此,我们使用 `r'<div class="comment">(.*?)</div>'` 来匹配这些评论。
最后,我们使用 `re.findall()` 函数来查找所有匹配的评论,并将它们输出到控制台。请注意,在这个例子中,我们使用了 re.DOTALL 标志,这是因为评论可能会跨越多行,而这个标志可以让点号(.)匹配所有字符,包括换行符。
相关问题
python爬虫正则表达式爬取网页数据
Python爬虫正则表达式爬取网页数据的方法如下:
1. 首先,需要导入所需的库:`requests`用于发送HTTP请求,`re`用于处理正则表达式。
```python
import requests
import re
```
2. 使用`requests.get()`方法获取网页内容。
```python
url = '目标网址'
response = requests.get(url)
content = response.text
```
3. 使用正则表达式提取所需数据。例如,提取所有的链接:
```python
pattern = r'<a href="(.*?)">'
links = re.findall(pattern, content)
```
4. 遍历并打印提取到的数据。
```python
for link in links:
print(link)
```
将以上代码整合在一起:
```python
import requests
import re
url = '目标网址'
response = requests.get(url)
content = response.text
pattern = r'<a href="(.*?)">'
links = re.findall(pattern, content)
for link in links:
print(link)
```
请将`目标网址`替换为实际要爬取的网址。
python爬虫正则表达式爬取图片练习题
好的,针对您的问题,我可以给您提供一个简单的爬取图片的示例代码:
```python
import re
import requests
url = 'https://www.example.com'
html = requests.get(url).text
img_urls = re.findall('img src="(.*?)"', html)
for img_url in img_urls:
img_data = requests.get(img_url).content
with open('image.jpg', 'wb') as f:
f.write(img_data)
```
这段代码会从指定的网站中爬取所有图片,并将其保存到本地。
阅读全文
相关推荐
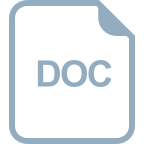
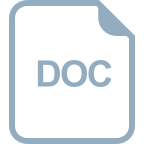
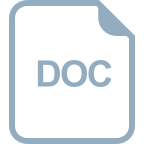
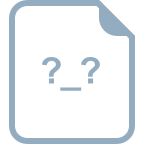
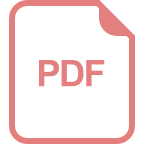









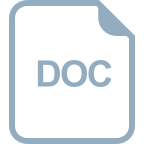