代码实现贪吃蛇游戏c++
时间: 2023-10-04 11:06:04 浏览: 110
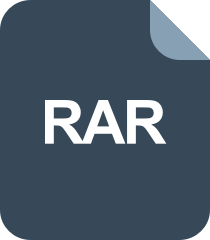
C++ 实现贪吃蛇游戏
以下是一个简单的贪吃蛇游戏的C++代码实现,使用了Windows API进行图形界面的绘制。
```c++
#include <iostream>
#include <windows.h>
using namespace std;
const int Width = 30; // 地图宽度
const int Height = 20; // 地图高度
int map[Height][Width] = {0}; // 地图,0表示空,1表示食物,2表示蛇身
struct Snake
{
int x;
int y;
Snake *next;
};
Snake *head, *tail; // 链表存储蛇身
int dir = 3; // 初始方向,1表示上,2表示左,3表示下,4表示右
void Init()
{
// 初始化蛇身
Snake *p = new Snake;
p->x = Width / 2;
p->y = Height / 2;
p->next = NULL;
head = tail = p;
map[p->y][p->x] = 2;
// 初始化食物
srand((unsigned)time(NULL));
int x = rand() % Width;
int y = rand() % Height;
while (map[y][x] != 0)
{
x = rand() % Width;
y = rand() % Height;
}
map[y][x] = 1;
}
void DrawMap()
{
HANDLE hOut;
hOut = GetStdHandle(STD_OUTPUT_HANDLE);
COORD pos;
pos.X = 0;
pos.Y = 0;
SetConsoleCursorPosition(hOut, pos);
for (int i = 0; i < Height; i++)
{
for (int j = 0; j < Width; j++)
{
if (map[i][j] == 0)
cout << " ";
else if (map[i][j] == 1)
cout << "@";
else
cout << "#";
}
cout << endl;
}
}
void Move()
{
int newx, newy;
newx = head->x;
newy = head->y;
if (dir == 1)
newy--;
else if (dir == 2)
newx--;
else if (dir == 3)
newy++;
else if (dir == 4)
newx++;
// 判断是否撞墙
if (newx < 0 || newx >= Width || newy < 0 || newy >= Height)
{
cout << "Game Over!" << endl;
exit(0);
}
// 判断是否撞到自己
if (map[newy][newx] == 2)
{
cout << "Game Over!" << endl;
exit(0);
}
// 判断是否吃到食物
if (map[newy][newx] == 1)
{
Snake *p = new Snake;
p->x = newx;
p->y = newy;
p->next = NULL;
tail->next = p;
tail = p;
map[newy][newx] = 2;
int x = rand() % Width;
int y = rand() % Height;
while (map[y][x] != 0)
{
x = rand() % Width;
y = rand() % Height;
}
map[y][x] = 1;
}
else
{
Snake *p = head;
head = head->next;
map[p->y][p->x] = 0;
p->x = newx;
p->y = newy;
map[newy][newx] = 2;
tail->next = p;
tail = p;
}
}
void Input()
{
if (_kbhit())
{
int key = _getch();
if (key == 72 && dir != 3)
dir = 1;
else if (key == 75 && dir != 4)
dir = 2;
else if (key == 80 && dir != 1)
dir = 3;
else if (key == 77 && dir != 2)
dir = 4;
}
}
int main()
{
Init();
while (true)
{
DrawMap();
Move();
Input();
Sleep(200);
}
return 0;
}
```
该代码实现了基本的贪吃蛇游戏功能,但是由于使用了Windows API进行图形界面的绘制,因此只能在Windows系统下运行。如果需要在其他系统下运行,需要修改图形界面的绘制方式。
阅读全文
相关推荐

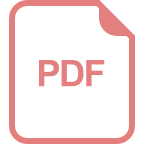
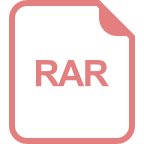
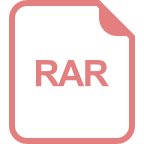
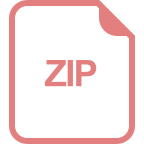
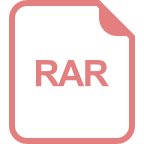
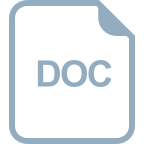
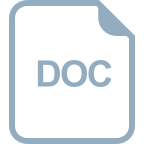
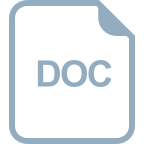
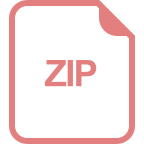
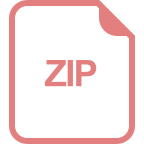