yolov5中detect文件中调用工业相机进行实时监测
时间: 2024-01-28 14:05:47 浏览: 30
要在YOLOv5的detect文件中调用工业相机进行实时监测,需要按照以下步骤进行操作:
1. 导入相应的工业相机SDK,并初始化相机。
2. 在循环中,不断获取相机的图像数据,并将其转换成YOLOv5所需的格式(如RGB或者灰度图像)。
3. 将转换后的图像数据输入到YOLOv5的模型中进行目标检测,获取检测结果。
4. 根据检测结果,对图像进行标注或者输出检测结果。
具体代码实现如下:
```
import cv2
import time
import torch
from models.experimental import attempt_load
from utils.datasets import LoadStreams, LoadImages
from utils.general import (check_img_size, non_max_suppression, apply_classifier, scale_coords, xyxy2xywh, plot_one_box, strip_optimizer, set_logging)
from utils.torch_utils import select_device, load_classifier, time_sync
# 导入工业相机SDK,例如Basler SDK
from pypylon import pylon
# 初始化相机
camera = pylon.InstantCamera(pylon.TlFactory.GetInstance().CreateFirstDevice())
camera.Open()
camera.StartGrabbing(pylon.GrabStrategy_LatestImageOnly)
# 加载YOLOv5模型
device = select_device('')
half = False # 是否使用半精度,根据模型大小和显存大小来定
model = attempt_load('yolov5s.pt', map_location=device)
imgsz = check_img_size(640, s=model.stride.max()) # 检查图片大小是否符合要求
if half:
model.half()
# 循环获取相机图像进行目标检测
while True:
# 获取相机图像
grabResult = camera.RetrieveResult(5000, pylon.TimeoutHandling_ThrowException)
img = grabResult.Array
# 转换图像格式
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# 图像预处理
img = cv2.resize(img, (imgsz[1], imgsz[0]))
img = torch.from_numpy(img).to(device)
img = img.half() if half else img.float()
img /= 255.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
# 目标检测
t1 = time_sync()
pred = model(img)[0]
# 后处理
pred = non_max_suppression(pred, 0.4, 0.5, classes=None, agnostic=False, max_det=1000)
t2 = time_sync()
# 显示结果
for i, det in enumerate(pred):
if len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], img.shape[2:]).round()
for *xyxy, conf, cls in reversed(det):
label = f'{model.names[int(cls)]} {conf:.2f}'
plot_one_box(xyxy, img0, label=label, color=colors[int(cls)], line_thickness=3)
# 显示图像
cv2.imshow('result', img)
cv2.waitKey(1)
# 释放相机图像缓存
grabResult.Release()
```
需要注意的是,在循环中调用相机进行实时监测时,需要实现图像的流畅传输,因此需要对代码进行优化,例如使用多线程或者多进程等方式来加速处理。同时,也需要根据相应的硬件设备和环境进行调整,以达到最佳的检测效果和性能表现。
相关推荐
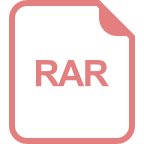
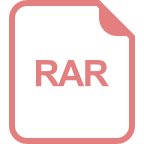














