#include<graphics.h> #define cube_size 20 //设置单个网格的大小 #define side 80 //设置一共有多少个网格 bool is_run = true; bool is_pause = false; bool field[side + 1][side + 1]; bool ass[side + 1][side + 1]; int d_x[3] = { -1,0,1 }; int d_y[3] = { -1,0,1 }; bool update(int x, int y) { int sum = 0; // 遍历9 格空间 for (int i = 0; i < 3; i++) for (int j = 0; j < 3; j++) { int xt = x + d_x[i], yt = y + d_y[j]; if (field[xt][yt]) sum += 1; } // 更新规则 if (field[x][y]) { if (sum < 3 || sum>4) return false; else return true; } else { if (sum == 3 || sum == 4) return true; else return false; } } int main() { initgraph(cube_size * side, cube_size * side); setbkcolor(BLACK); cleardevice(); setfillcolor(GREEN); setlinecolor(WHITE); for (int i = 0; i < side; i++) { line(i * cube_size, 0, i * cube_size, side * cube_size); line(0, i * cube_size, side * cube_size, i * cube_size);; } while (is_run) { Sleep(500); // 画图 for (int i = 0; i < side; i++) for (int j = 0; j < side; j++) { if (field[i][j]) fillrectangle(i * cube_size, j * cube_size, (i + 1) * cube_size, (j + 1) * cube_size); else clearrectangle(i * cube_size + 1, j * cube_size + 1, (i + 1) * cube_size - 1, (j + 1) * cube_size - 1); }
时间: 2024-02-14 14:21:35 浏览: 126
这段代码实现了一个经典的生命游戏(Conway's Game of Life)的模拟。其中,定义了一个80*80的方格状的生命游戏世界,每个方格的大小为20*20像素。代码中使用了两个bool类型的数组来表示当前状态和下一个状态的生命情况,通过遍历每个方格周围的8个方格来更新每个方格的状态,最后使用图形库绘制出了生命游戏的模拟效果。
相关问题
#define _CRT_SECURE_NO_WARNINGS #include <stdio.h> #include <stdlib.h> #include <string.h> #define PHONE_SIZE 50 #define NAME_SIZE 20 typedef struct lxr { char phone[PHONE_SIZE]; char name[NAME_SIZE]; }lxr; int main() { lxr a[10]; a[0].phone = "liujunjie"; printf("%s", a[0].phone); return 0; }
这段代码存在一些问题。首先,您不能直接将字符串赋值给字符数组。其次,如果您想将字符串复制到字符数组中,您应该使用strcpy函数而不是直接赋值运算符。另外,还需要注意将字符串赋值给字符数组时,字符数组的大小应该足够大以容纳整个字符串。以下是修改后的代码:
```c
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define PHONE_SIZE 50
#define NAME_SIZE 20
typedef struct lxr {
char phone[PHONE_SIZE];
char name[NAME_SIZE];
} lxr;
int main()
{
lxr a[10];
strcpy(a[0].phone, "liujunjie"); // 使用strcpy函数将字符串复制给字符数组
printf("%s", a[0].phone);
return 0;
}
```
这样,代码就能正常运行并输出"liujunjie"。
补充以下代码 #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <stdlib.h> #include <stdio.h> #define BUFFER_SIZE 1024 //请不要更改文件路径!!! #define SRC_FILE_NAME "/data/workspace/myshixun/fileSystem/src/fileProgram/src_file" //请不要更改文件路径!!! #define DEST_FILE_NAME "dest_file" #define OFFSET 10240 int main() { int src_file,dest_file; unsigned char buff[BUFFER_SIZE]; int real_read_len; //请在此处填入代码,使用合适的模式打开源目标SRC_FILE_NAME文件 src_file = //请在此处填入代码,使用合适的模式打开写入文件目标DEST_FILE_NAME文件,需要考虑到文件是否存在? dest_file = if(src_file < 0 || dest_file < 0) { printf("Open file error!\n"); exit(1); } //请在此处填入代码,设置偏移量读取文件最后10KB数据 while((real_read_len = read(src_file,buff,sizeof(buff))) > 0) { //请在此处填入代码,使用buff写入目标文件 } close(dest_file); close(src_file); return 0; }
补充的代码如下:
```
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdlib.h>
#include <stdio.h>
#define BUFFER_SIZE 1024
#define SRC_FILE_NAME "/data/workspace/myshixun/fileSystem/src/fileProgram/src_file"
#define DEST_FILE_NAME "dest_file"
#define OFFSET 10240
int main() {
int src_file, dest_file;
unsigned char buff[BUFFER_SIZE];
int real_read_len;
// 打开源文件
src_file = open(SRC_FILE_NAME, O_RDONLY);
// 打开目标文件,如果文件不存在则创建它,如果存在则清空内容
dest_file = open(DEST_FILE_NAME, O_WRONLY | O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR);
if (src_file < 0 || dest_file < 0) {
printf("Open file error!\n");
exit(1);
}
// 设置偏移量读取文件最后10KB数据
lseek(src_file, -OFFSET, SEEK_END);
while ((real_read_len = read(src_file, buff, sizeof(buff))) > 0) {
// 使用 buff 写入目标文件
write(dest_file, buff, real_read_len);
}
close(dest_file);
close(src_file);
return 0;
}
```
此程序的功能是从文件的最后10KB处读取数据,并将其写入到另一个文件中。其中,SRC_FILE_NAME 是源文件的路径,DEST_FILE_NAME 是目标文件的路径,OFFSET 是偏移量,表示从文件末尾往前偏移的字节数。程序中使用了系统调用 open、read、write 和 lseek 来实现文件的读写操作。
阅读全文
相关推荐
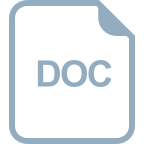
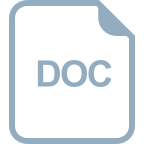
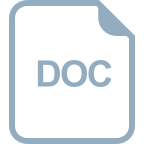



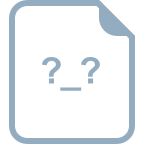
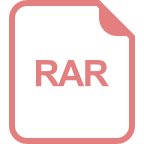
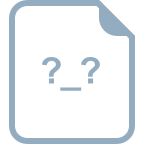
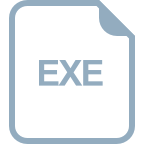
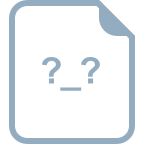
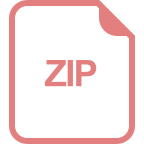
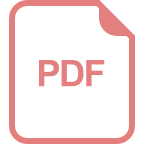
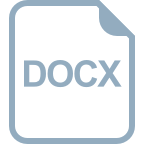
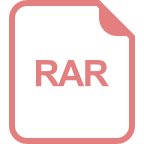
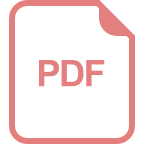
