vue实现左边下拉框右边输入框并校验
时间: 2023-09-04 11:06:33 浏览: 59
下面是一个简单的实现示例:
```
<template>
<div class="form-group">
<label>{{ label }}</label>
<div class="form-control">
<select v-model="selectedItem" @change="validateInput">
<option value="">请选择</option>
<option v-for="item in options" :key="item.value" :value="item.value">{{ item.label }}</option>
</select>
<input v-model="inputValue" @input="validateInput" />
<div class="error-message">{{ errorMessage }}</div>
</div>
</div>
</template>
<script>
export default {
props: {
label: {
type: String,
required: true
},
options: {
type: Array,
required: true
},
validate: {
type: Function,
required: true
}
},
data() {
return {
selectedItem: '',
inputValue: '',
errorMessage: ''
}
},
methods: {
validateInput() {
const isValid = this.validate(this.selectedItem, this.inputValue)
this.errorMessage = isValid ? '' : '输入格式不正确'
}
}
}
</script>
<style>
.form-group {
margin-bottom: 20px;
}
.form-control {
display: flex;
align-items: center;
}
.form-control select {
margin-right: 5px;
}
.error-message {
color: red;
font-size: 12px;
margin-top: 5px;
}
</style>
```
在父组件中,你可以这样使用它:
```
<template>
<div>
<InputWithSelect
label="姓名"
:options="nameOptions"
:validate="validateName"
/>
</div>
</template>
<script>
import InputWithSelect from './InputWithSelect.vue'
export default {
components: {
InputWithSelect
},
data() {
return {
nameOptions: [
{ label: '张三', value: 'zhangsan' },
{ label: '李四', value: 'lisi' },
{ label: '王五', value: 'wangwu' }
]
}
},
methods: {
validateName(selectedItem, inputValue) {
return /^[a-zA-Z]+$/.test(inputValue)
}
}
}
</script>
```
如上代码所示,你需要传递一个选项数组、一个验证函数以及一个标签字符串给 `InputWithSelect` 组件。选项数组包含下拉框中的选项,验证函数接收两个参数(下拉框中的选项和输入框中的值),并返回一个布尔值表示是否通过验证。在子组件中,我们可以通过 `v-model` 指令来双向绑定下拉框和输入框的值,并在 `validateInput` 方法中调用验证函数,更新错误信息。最后,我们在模板中显示错误信息。
相关推荐
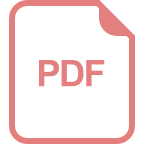
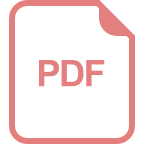











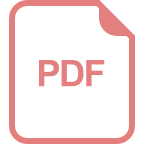
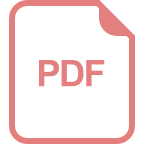
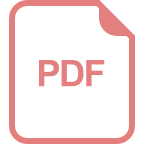
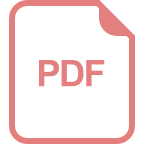
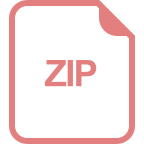