一个线性表有n个元素(n<MAXSIZE, MAXSIZE指线性表的最大长度)且递增。现有一元素x要插入到线性表的适当位置上,并保持线性表原有的顺序不变。设计程序实现。 要求:(1)采用顺序存储表示实现;(2)线性表元素分别用输入来给出、用随机数来给出;
时间: 2024-09-13 20:14:03 浏览: 39
为了实现这个功能,你可以按照以下步骤编写程序:
1. **初始化线性表**:首先,创建一个数组或动态数组来存储线性表的元素,假设其类型为`T`。由于`n < MAXSIZE`,所以初始化数组大小为`MAXSIZE`。
```c++
T linearList[MAXSIZE];
int n = 0; // 线性表当前元素的数量
```
2. **读取元素并填充线性表**:如果所有元素已给定,则直接将它们按递增顺序插入数组。如果需要生成随机数作为元素,可以先读入前`n`个元素,然后使用`rand()`函数生成第`n+1`个元素的值,确保它是递增的。
```c++
// 如果元素给定
for (int i = 0; i < n; i++) {
linearList[i] = input[i]; // 输入元素
}
// 如果元素是随机的
if (generateRandom) {
T x = generateRandomValue();
for (int i = n - 1; i >= 0 && x > linearList[i]; i--) {
linearList[i + 1] = linearList[i];
}
linearList[i + 1] = x;
}
```
3. **找到插入位置**:遍历数组以查找元素`x`应该插入的位置,即找到第一个大于`x`的元素的索引。这一步可以用`binary_search`或从后向前遍历数组直到找到合适的位置。
```c++
int insertIndex = n; // 假设x比所有现有元素都大
for (int i = n - 1; i >= 0 && linearList[i] <= x; i--) {
insertIndex--;
}
// 插入操作
linearList[insertIndex] = x;
```
4. **更新元素计数**:最后,增加线性表的实际元素数量`n`。
```c++
n++;
```
完整的伪代码示例如下:
```c++
function insertIntoLinearList(x):
T[] linearList = new T[MAXSIZE]
int n = 0
// ... (读取/生成元素)
// 查找插入位置
int insertIndex = n
for (int i = n - 1 to 0):
if linearList[i] > x:
insertIndex = i
break
// 插入元素
linearList[insertIndex] = x
n++
return linearList, n
```
阅读全文
相关推荐
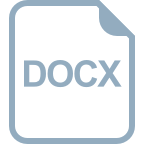
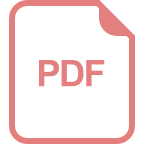
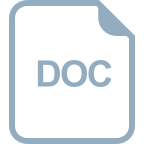











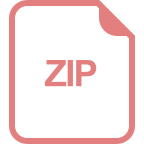