用Python的tkinter写出俄罗斯方块游戏
时间: 2023-11-19 14:09:07 浏览: 117
好的,下面是一个简单的俄罗斯方块游戏的代码,使用Python的tkinter模块实现。
```python
import tkinter as tk
import random
# 方块的种类和颜色
shapes = [
[[1, 1, 1], [0, 1, 0]], # T
[[1, 1, 1, 1]], # I
[[1, 1, 0], [0, 1, 1]], # Z
[[0, 1, 1], [1, 1, 0]], # S
[[1, 1], [1, 1]], # O
[[1, 1, 1], [1, 0, 0]], # J
[[1, 1, 1], [0, 0, 1]] # L
]
colors = ["#ff0000", "#00ff00", "#0000ff", "#ffff00", "#00ffff", "#ff00ff", "#ffa500"]
class Tetris:
def __init__(self):
self.width = 10
self.height = 20
self.score = 0
self.level = 1
self.delay = 500
self.game_over = False
self.timer = None
self.root = tk.Tk()
self.root.title("俄罗斯方块")
self.root.resizable(False, False)
self.canvas = tk.Canvas(self.root, width=300, height=600, borderwidth=0, highlightthickness=0)
self.canvas.pack(padx=10, pady=10)
self.info_panel = tk.Frame(self.root, width=100, height=600, bg="white")
self.info_panel.pack(side="left", padx=(0, 10), pady=10)
tk.Label(self.info_panel, text="得分").pack(pady=(10, 0))
self.score_label = tk.Label(self.info_panel, text="0")
self.score_label.pack()
tk.Label(self.info_panel, text="级别").pack(pady=(10, 0))
self.level_label = tk.Label(self.info_panel, text="1")
self.level_label.pack()
tk.Label(self.info_panel, text="下一个方块").pack(pady=(10, 0))
self.next_canvas = tk.Canvas(self.info_panel, width=80, height=80, borderwidth=0, highlightthickness=0)
self.next_canvas.pack()
self.draw_next_shape()
self.init_board()
self.root.bind("<Key>", self.key_pressed)
def init_board(self):
self.board = [[0 for _ in range(self.width)] for _ in range(self.height)]
self.current_shape = self.get_new_shape()
self.next_shape = self.get_new_shape()
self.draw_board()
def draw_board(self):
self.canvas.delete("all")
for y in range(self.height):
for x in range(self.width):
if self.board[y][x] != 0:
color = colors[self.board[y][x] - 1]
self.canvas.create_rectangle(x * 30, y * 30, (x + 1) * 30, (y + 1) * 30, fill=color, outline="")
for y in range(len(self.current_shape)):
for x in range(len(self.current_shape[0])):
if self.current_shape[y][x] != 0:
color = colors[self.current_shape[y][x] - 1]
self.canvas.create_rectangle((self.current_x + x) * 30, (self.current_y + y) * 30,
(self.current_x + x + 1) * 30, (self.current_y + y + 1) * 30,
fill=color, outline="")
def key_pressed(self, event):
if event.keysym == "Left":
self.move_left()
elif event.keysym == "Right":
self.move_right()
elif event.keysym == "Down":
self.move_down()
elif event.keysym == "Up":
self.rotate()
def move_left(self):
if not self.game_over:
if self.check_collision(self.current_shape, self.current_x - 1, self.current_y):
self.current_x -= 1
self.draw_board()
def move_right(self):
if not self.game_over:
if self.check_collision(self.current_shape, self.current_x + 1, self.current_y):
self.current_x += 1
self.draw_board()
def move_down(self):
if not self.game_over:
if self.check_collision(self.current_shape, self.current_x, self.current_y + 1):
self.current_y += 1
self.draw_board()
else:
self.freeze_shape()
self.clear_lines()
self.current_shape = self.next_shape
self.next_shape = self.get_new_shape()
self.draw_next_shape()
self.current_x = self.width // 2 - len(self.current_shape[0]) // 2
self.current_y = 0
if not self.check_collision(self.current_shape, self.current_x, self.current_y):
self.game_over = True
self.canvas.create_text(150, 300, text="游戏结束", font=("Arial", 32), fill="red")
def rotate(self):
if not self.game_over:
rotated_shape = [[self.current_shape[y][x] for y in range(len(self.current_shape))] for x in range(len(self.current_shape[0]) - 1, -1, -1)]
if self.check_collision(rotated_shape, self.current_x, self.current_y):
self.current_shape = rotated_shape
self.draw_board()
def freeze_shape(self):
for y in range(len(self.current_shape)):
for x in range(len(self.current_shape[0])):
if self.current_shape[y][x] != 0:
self.board[self.current_y + y][self.current_x + x] = self.current_shape[y][x]
def clear_lines(self):
lines_cleared = 0
for y in range(self.height):
if all(self.board[y]):
lines_cleared += 1
del self.board[y]
self.board.insert(0, [0 for _ in range(self.width)])
if lines_cleared > 0:
self.score += lines_cleared ** 2 * 10
self.score_label.config(text=str(self.score))
self.level = 1 + self.score // 100
self.level_label.config(text=str(self.level))
self.delay = int(500 / self.level)
self.root.after_cancel(self.timer)
self.timer = self.root.after(self.delay, self.move_down)
def get_new_shape(self):
return random.choice(shapes)
def draw_next_shape(self):
self.next_canvas.delete("all")
shape = self.next_shape
for y in range(len(shape)):
for x in range(len(shape[0])):
if shape[y][x] != 0:
color = colors[shape[y][x] - 1]
self.next_canvas.create_rectangle(x * 20, y * 20, (x + 1) * 20, (y + 1) * 20, fill=color, outline="")
def check_collision(self, shape, x, y):
for cy in range(len(shape)):
for cx in range(len(shape[0])):
if shape[cy][cx] != 0:
try:
if self.board[y + cy][x + cx] != 0:
return False
except IndexError:
return False
return True
def run(self):
self.timer = self.root.after(self.delay, self.move_down)
self.root.mainloop()
if __name__ == "__main__":
game = Tetris()
game.run()
```
运行代码后,会出现一个带有游戏界面和信息面板的窗口。使用方向键控制方块的移动和旋转,当一行被填满时会消除,得分和级别会相应地增加。如果方块到达顶部就会游戏结束。
阅读全文
相关推荐
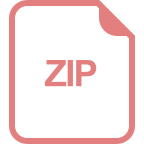
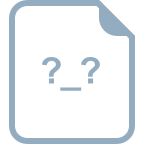
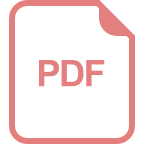
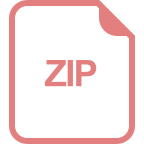





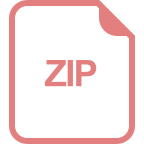
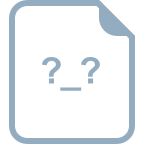
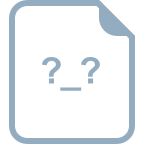
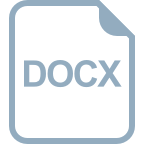
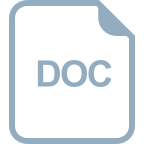
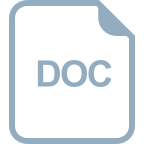



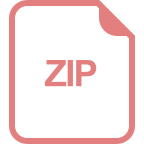