Java代码配置IBMmq的队列
时间: 2024-03-15 08:47:28 浏览: 15
以下是使用 Java 代码配置 IBM MQ 队列的示例:
```java
import com.ibm.mq.*;
public class MQQueueManagerExample {
private MQQueueManager queueManager;
public MQQueueManagerExample(String queueManagerName, String hostName, int port, String channelName) throws MQException {
MQEnvironment.hostname = hostName;
MQEnvironment.port = port;
MQEnvironment.channel = channelName;
MQEnvironment.userID = "myUser";
MQEnvironment.password = "myPassword";
this.queueManager = new MQQueueManager(queueManagerName);
}
public void createQueue(String queueName) throws MQException {
int openOptions = MQConstants.MQOO_OUTPUT | MQConstants.MQOO_FAIL_IF_QUIESCING;
MQQueue queue = this.queueManager.accessQueue(queueName, openOptions);
queue.close();
}
public void close() {
if (this.queueManager != null) {
try {
this.queueManager.close();
} catch (MQException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
String queueManagerName = "myQueueManager";
String hostName = "localhost";
int port = 1414;
String channelName = "myChannel";
String queueName = "myQueue";
try {
MQQueueManagerExample example = new MQQueueManagerExample(queueManagerName, hostName, port, channelName);
example.createQueue(queueName);
example.close();
System.out.println("Queue created successfully.");
} catch (MQException e) {
e.printStackTrace();
}
}
}
```
以上示例代码中,我们使用 `MQQueueManager` 类创建了一个队列管理器实例,并通过设置 `MQEnvironment` 类的静态属性来设置 IBM MQ 连接参数。接着,我们使用 `accessQueue` 方法创建了一个队列,并通过设置 `openOptions` 参数来指定队列的属性。最后,在队列创建成功后,我们通过 `close` 方法关闭了队列管理器实例。
需要注意的是,上述示例代码中的连接参数、队列管理器名称、队列名称等信息需要根据实际情况进行修改。此外,在创建队列时,可以根据需要设置更多的属性,例如队列类型、最大深度、最大消息长度等。
相关推荐
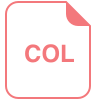
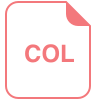
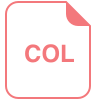











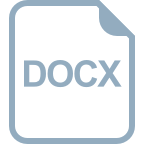