C语言链表实现N皇后
时间: 2024-12-20 16:04:32 浏览: 3
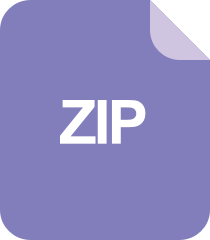
利用C语言用栈求解n皇后问题的代码
好的,我很乐意为您介绍如何使用C语言链表实现N皇后问题。
N皇后问题是经典的回溯算法应用,使用链表可以有效地存储和操作棋盘状态。以下是使用C语言链表实现N皇后问题的主要步骤:
1. 定义链表节点结构:
```c
typedef struct Node {
int *board;
int queens;
struct Node *next;
} Node;
```
2. 初始化链表:
```c
Node *createNode(int n) {
Node *node = (Node *)malloc(sizeof(Node));
node->board = (int *)calloc(n, sizeof(int));
node->queens = 0;
node->next = NULL;
return node;
}
```
3. 添加新节点到链表:
```c
void addNode(Node **head, int *board, int queens) {
Node *newNode = createNode(queens);
for (int i = 0; i < queens; i++) {
newNode->board[i] = board[i];
}
newNode->queens = queens;
newNode->next = *head;
*head = newNode;
}
```
4. 检查位置是否安全:
```c
int isSafe(int *board, int row, int col, int n) {
for (int i = 0; i < row; i++) {
if (board[i] == col ||
abs(board[i] - col) == abs(i - row)) {
return 0;
}
}
return 1;
}
```
5. 回溯函数:
```c
void solveNQueens(Node **solutions, int *board, int row, int n) {
if (row == n) {
addNode(solutions, board, n);
return;
}
for (int col = 0; col < n; col++) {
if (isSafe(board, row, col, n)) {
board[row] = col;
solveNQueens(solutions, board, row + 1, n);
}
}
}
```
6. 主函数:
```c
int main() {
int n;
printf("Enter the value of N for N-Queens problem: ");
scanf("%d", &n);
Node *solutions = NULL;
int *board = (int *)calloc(n, sizeof(int));
solveNQueens(&solutions, board, 0, n);
printf("Total solutions: %d\n", countNodes(solutions));
// 打印所有解
printSolutions(solutions, n);
// 释放内存
free(board);
freeLinkedList(solutions);
return 0;
}
```
这个实现使用链表来存储所有可能的解。每次找到一个有效解时,就将其添加到链表中。最后,程序会打印出总解数和所有具体的解法。
使用链表的好处是它允许动态地添加和删除元素,这对于存储变长度的解集特别有用。同时,链表的实现使得我们能够在找到解的过程中就立即存储它们,而不需要预先知道解的总数。
阅读全文
相关推荐
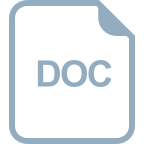
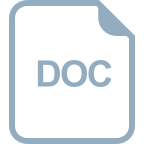
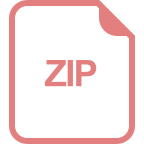
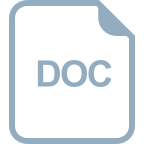
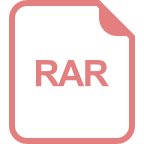
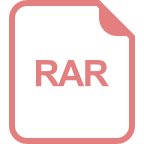
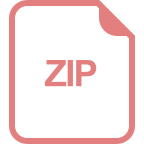
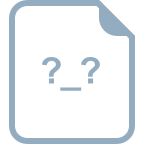
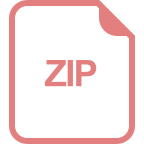
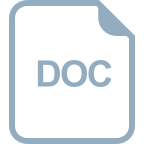
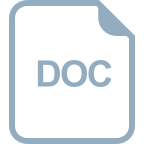
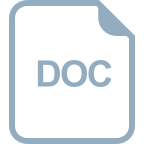
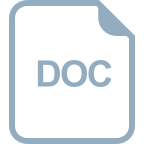
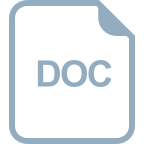
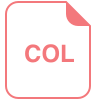
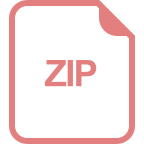