算法实现与应用:C语言中实现经典算法的案例分析
发布时间: 2024-12-12 08:56:26 阅读量: 9 订阅数: 15 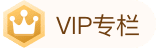
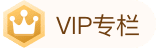
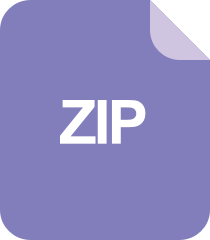
数据结构与算法分析:C语言描述_数据结构与算法分析图书_

# 1. C语言与经典算法概述
在计算机科学与技术领域,C语言一直以来都是学习和应用的基础。它以其接近硬件的特性、高效的执行能力和灵活的操作性,在系统编程、嵌入式开发以及跨平台软件开发中占有举足轻重的地位。掌握C语言不仅是IT专业人员的基本技能,也是深入理解计算机工作原理的重要途径。
经典算法是指那些在时间的检验中被证明既高效又实用的算法。它们通常具有严谨的理论基础,并广泛应用于软件开发、数据分析、人工智能等多个领域。学习经典算法能够帮助我们构建强大的解决问题的工具集,提升开发效率和软件质量。
本章首先将带您回顾C语言的基础知识,包括其语法特性和编程范式。接着,我们探讨几个基础但至关重要的经典算法,如排序和搜索算法,并阐述其在C语言中的实现方法。通过本章,读者将为深入理解数据结构和更复杂的算法打下坚实的基础。
# 2. 数据结构基础与算法实现
### 2.1 线性结构与算法实现
#### 2.1.1 数组和链表的基本操作
数组和链表是线性数据结构中最基本也是最重要的两种结构,它们各有优劣,被广泛应用于各种算法实现中。
**数组**是一种线性数据结构,它可以存储固定大小的同类型元素。数组中的每个元素可以通过下标直接访问,下标从0开始。由于数组在内存中是连续存储的,因此访问速度快,但是在插入或删除元素时,需要移动大量元素,效率较低。
**链表**由一系列节点组成,每个节点包含数据域和指向下一个节点的指针。链表不一定要在内存中连续存储,因此在插入或删除操作时只需要改变相关节点的指针即可,操作效率高,但是访问效率低,因为必须从头节点开始,沿着链表顺序查找。
下面是一个简单的链表节点定义和基本操作的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 数据域
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建链表节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation error!\n");
exit(1);
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 在链表尾部插入节点
void insertAtEnd(Node** head, int data) {
Node* newNode = createNode(data);
if (*head == NULL) {
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 打印链表
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
// 释放链表内存
void freeList(Node* head) {
Node* temp;
while (head != NULL) {
temp = head;
head = head->next;
free(temp);
}
}
int main() {
Node* head = NULL; // 创建一个空链表
insertAtEnd(&head, 1);
insertAtEnd(&head, 2);
insertAtEnd(&head, 3);
printList(head);
freeList(head);
return 0;
}
```
在上述代码中,定义了一个链表节点结构体,以及创建新节点、在链表尾部插入新节点、打印链表和释放链表内存的操作函数。通过这些基本操作,我们可以构建和管理链表数据结构。
#### 2.1.2 栈和队列的算法应用
**栈**是一种后进先出(LIFO)的数据结构,它有两个基本操作:push(进栈)和pop(出栈)。栈的主要用途之一是实现函数调用的维护,它也可以用于解决诸如括号匹配、表达式求值、深度优先搜索等算法问题。
**队列**是一种先进先出(FIFO)的数据结构,基本操作有enqueue(入队)和dequeue(出队)。队列在算法中经常用于实现广度优先搜索、打印任务的管理等。
下面是一个栈和队列的基本实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 5
// 栈结构定义
typedef struct Stack {
int top;
int data[MAXSIZE];
} Stack;
// 初始化栈
void initStack(Stack* s) {
s->top = -1;
}
// 判断栈是否为空
int isEmpty(Stack* s) {
return s->top == -1;
}
// 判断栈是否已满
int isFull(Stack* s) {
return s->top == MAXSIZE - 1;
}
// 进栈操作
void push(Stack* s, int value) {
if (isFull(s)) {
printf("Stack is full!\n");
return;
}
s->data[++s->top] = value;
}
// 出栈操作
int pop(Stack* s) {
if (isEmpty(s)) {
printf("Stack is empty!\n");
return -1;
}
return s->data[s->top--];
}
// 队列结构定义
typedef struct Queue {
int front, rear;
int data[MAXSIZE];
} Queue;
// 初始化队列
void initQueue(Queue* q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
int isQueueEmpty(Queue* q) {
return q->front == q->rear;
}
// 进队操作
void enqueue(Queue* q, int value) {
if (q->rear == MAXSIZE) {
printf("Queue is full!\n");
return;
}
q->data[q->rear++] = value;
}
// 出队操作
int dequeue(Queue* q) {
if (isQueueEmpty(q)) {
printf("Queue is empty!\n");
return -1;
}
return q->data[q->front++];
}
int main() {
Stack s;
initStack(&s);
push(&s, 1);
push(&s, 2);
push(&s, 3);
printf("Popped element: %d\n", pop(&s)); // 输出: Popped element: 3
printf("Popped element: %d\n", pop(&s)); // 输出: Popped element: 2
Queue q;
initQueue(&q);
enqueue(&q, 1);
enqueue(&q, 2);
enqueue(&q, 3);
printf("Dequeued element: %d\n", dequeue(&q)); // 输出
```
0
0
相关推荐
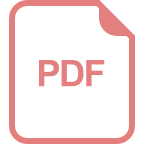
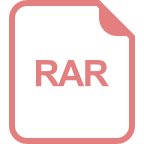
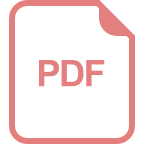
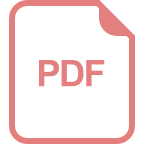
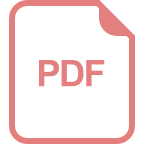
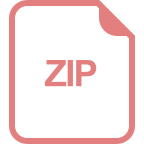
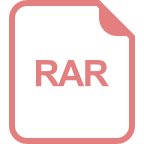
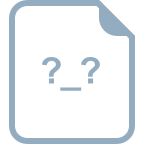