Python 在爬取网页代码出现乱码怎么解决
时间: 2023-06-17 08:08:03 浏览: 177
在爬取网页时出现乱码,可能是因为网页的编码与 Python 默认的编码不一致导致的。下面提供几种解决方法:
1. 使用 chardet 库自动检测网页编码:
```python
import requests
import chardet
url = "http://example.com"
response = requests.get(url)
encoding = chardet.detect(response.content)['encoding']
html = response.content.decode(encoding)
```
2. 指定编码进行解码:
```python
import requests
url = "http://example.com"
response = requests.get(url)
response.encoding = "utf-8"
html = response.text
```
3. 使用 BeautifulSoup 库解析网页:
```python
import requests
from bs4 import BeautifulSoup
url = "http://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
```
在使用 BeautifulSoup 解析时,它会自动识别网页编码并解码,因此可以避免编码问题。
相关问题
python爬取的网页出现乱码
出现乱码的原因可能是网页编码与你所使用的编码不一致,可以尝试使用 chardet 库来自动检测网页编码,然后再使用相应的编码进行解码。具体操作可以参考以下代码:
```python
import requests
import chardet
url = 'http://www.example.com'
response = requests.get(url)
encoding = chardet.detect(response.content)['encoding']
html = response.content.decode(encoding)
```
其中,`chardet.detect(response.content)['encoding']` 用于检测网页编码,`response.content.decode(encoding)` 用于解码网页内容。
python爬取网页出现乱码
出现乱码的原因可能是网页编码与你所使用的编码不一致,可以尝试使用 chardet 库来自动检测网页编码,然后再进行解码。具体操作可以参考以下代码:
```python
import requests
import chardet
url = 'https://www.example.com'
response = requests.get(url)
encoding = chardet.detect(response.content)['encoding']
html = response.content.decode(encoding)
```
其中,`chardet.detect()` 函数可以自动检测编码,返回一个字典,其中 'encoding' 键对应的值即为检测到的编码。
阅读全文
相关推荐
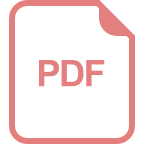
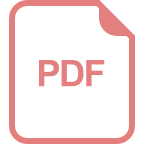
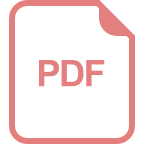


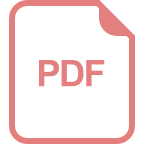
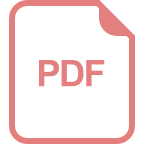
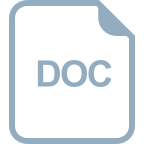







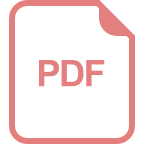