MapReduce编程模型中,主要由哪三个组件构成?同时,请提供一个典型的Main函数在MapReduce程序中的基本结构代码示例。
时间: 2024-12-16 16:19:08 浏览: 7
MapReduce编程模型主要由三个核心组件组成:
1. **Mapper**:负责对原始数据进行处理,将输入的数据分成一个个独立的片段(键值对),并对每个片段应用用户自定义的map函数进行转换。
2. **Reducer**:接收Mapper处理后的中间结果,对具有相同键的值进行聚合,生成最终的结果键值对。
3. **Shuffle/Sort**:这是两个过程,首先Mapper产生的中间结果会通过网络进行shuffle,然后Reducer接收到数据后,按照键进行排序后再进行reduce操作。
下面是一个简单的Hadoop Java MapReduce程序的`Main`函数的基本结构代码示例:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class WordCount {
public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> {
// map函数的具体实现...
@Override
protected void map(Object key, Text value, Context context) throws IOException, InterruptedException {
// 分词并发送到reducer
}
}
public static class IntSumReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
// reduce函数的具体实现...
@Override
protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
context.write(key, new IntWritable(sum));
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(WordCount.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(IntSumReducer.class); // 可选设置,通常用于优化
job.setReducerClass(IntSumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在这个例子中,`TokenizerMapper`负责读取文本文件,分词,并将单词映射到`Text`键和`1`值;`IntSumReducer`接收键值对,累加每个单词出现的次数。最后,`main`函数配置了任务的输入、输出路径等信息,并启动任务运行。
阅读全文
相关推荐
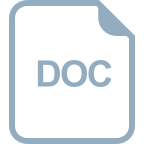
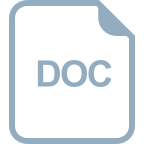
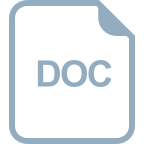
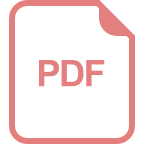
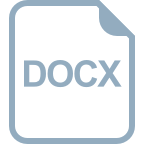
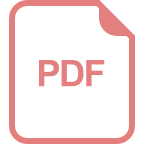
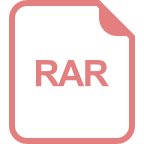
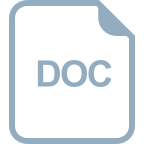
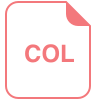
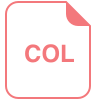
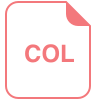
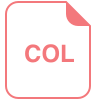
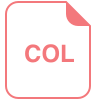
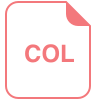
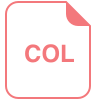
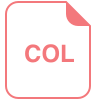
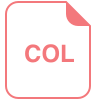
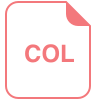
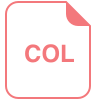