写一份基于Python的图书管理系统的程序
时间: 2024-02-25 15:57:16 浏览: 15
好的,下面是一个基于Python的图书管理系统的程序,主要实现了图书的增删改查功能:
```python
import sqlite3
# 连接数据库
conn = sqlite3.connect('books.db')
c = conn.cursor()
# 创建表格
c.execute('''CREATE TABLE IF NOT EXISTS books
(id INTEGER PRIMARY KEY AUTOINCREMENT,
title TEXT NOT NULL,
author TEXT NOT NULL,
publisher TEXT NOT NULL,
pub_date DATE NOT NULL,
isbn TEXT NOT NULL)''')
# 添加图书
def add_book(title, author, publisher, pub_date, isbn):
c.execute("INSERT INTO books (title, author, publisher, pub_date, isbn) VALUES (?, ?, ?, ?, ?)",
(title, author, publisher, pub_date, isbn))
conn.commit()
print("Add book successfully.")
# 删除图书
def delete_book(book_id):
c.execute("DELETE FROM books WHERE id=?", (book_id,))
conn.commit()
print("Delete book successfully.")
# 更新图书
def update_book(book_id, title, author, publisher, pub_date, isbn):
c.execute("UPDATE books SET title=?, author=?, publisher=?, pub_date=?, isbn=? WHERE id=?",
(title, author, publisher, pub_date, isbn, book_id))
conn.commit()
print("Update book successfully.")
# 查询图书
def search_book(title=None, author=None, publisher=None, pub_date=None, isbn=None):
if title is None and author is None and publisher is None and pub_date is None and isbn is None:
c.execute("SELECT * FROM books")
else:
query = "SELECT * FROM books WHERE"
if title is not None:
query += " title='" + title + "' AND"
if author is not None:
query += " author='" + author + "' AND"
if publisher is not None:
query += " publisher='" + publisher + "' AND"
if pub_date is not None:
query += " pub_date='" + pub_date + "' AND"
if isbn is not None:
query += " isbn='" + isbn + "' AND"
query = query[:-4] # 去掉最后一个AND
c.execute(query)
books = c.fetchall()
for book in books:
print(book)
# 测试
add_book("Python入门教程", "张三", "清华大学出版社", "2021-01-01", "978-7-302-xxx-x")
add_book("数据结构与算法", "李四", "人民邮电出版社", "2020-12-01", "978-7-115-xxx-x")
search_book(title="Python入门教程")
update_book(1, "Python基础教程", "张三", "清华大学出版社", "2021-01-01", "978-7-302-xxx-x")
search_book(title="Python基础教程")
delete_book(2)
search_book()
```
该程序使用了SQLite作为数据库,通过调用SQL语句来实现增删改查等功能。你可以根据实际需要进行更改和扩展。
相关推荐
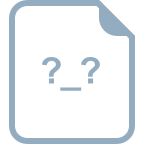
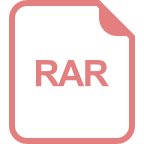
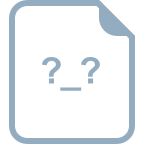














