QT中将BMP图片并将值写到另一个txt文件中
时间: 2023-09-11 17:06:52 浏览: 96
要将BMP图片读取并将值写入另一个txt文件,可以使用QT中提供的QImage和QFile类。
以下是一个简单的示例代码,可以将BMP图片的像素值写入txt文件中:
```cpp
#include <QCoreApplication>
#include <QImage>
#include <QFile>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 读取BMP图片
QImage image("test.bmp");
if (image.isNull()) {
qDebug() << "Failed to load image!";
return -1;
}
// 打开txt文件
QFile file("output.txt");
if (!file.open(QIODevice::WriteOnly | QIODevice::Text)) {
qDebug() << "Failed to open file!";
return -1;
}
// 将像素值写入txt文件
QTextStream out(&file);
for (int y = 0; y < image.height(); y++) {
for (int x = 0; x < image.width(); x++) {
QColor color = image.pixelColor(x, y);
out << color.red() << " " << color.green() << " " << color.blue() << "\t";
}
out << "\n";
}
// 关闭文件
file.close();
qDebug() << "Done!";
return a.exec();
}
```
该程序首先使用QImage类读取了名为"test.bmp"的BMP图片,然后使用QFile类打开了名为"output.txt"的txt文件。接着,使用QTextStream类将图片中每个像素的红、绿、蓝三个通道的值写入txt文件中。最后,关闭文件并输出完成提示。
请注意,这只是一个简单的示例程序,可能无法处理所有情况。例如,如果BMP图片的位深度不是24位,则可能需要使用不同的方法来处理像素值。
阅读全文
相关推荐
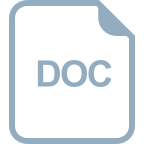
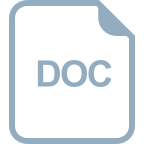
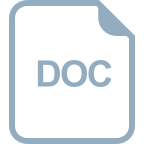







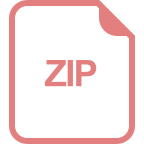
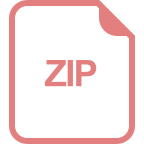
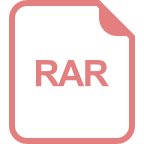
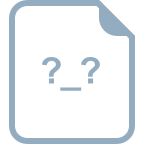
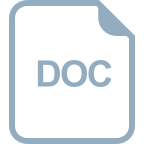
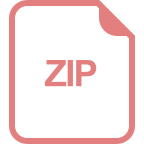
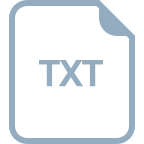
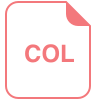
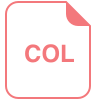