android线程倒计时,Android Thread线程倒计时卡顿-postDelayed/runOnUiThread/AsyncTask
时间: 2024-01-25 11:02:20 浏览: 25
在Android中,线程倒计时可以通过多种方式实现,包括使用postDelayed、runOnUiThread和AsyncTask。
1. 使用postDelayed方式实现线程倒计时:
```
final Handler handler = new Handler();
handler.postDelayed(new Runnable() {
int count = 10;
@Override
public void run() {
// 更新UI
tvCountDown.setText("" + count);
count--;
if (count >= 0) {
handler.postDelayed(this, 1000); // 一秒后再次执行
} else {
// 倒计时结束
}
}
}, 1000); // 延迟一秒后执行
```
2. 使用runOnUiThread方式实现线程倒计时:
```
new Thread(new Runnable() {
int count = 10;
@Override
public void run() {
while (count >= 0) {
runOnUiThread(new Runnable() {
@Override
public void run() {
// 更新UI
tvCountDown.setText("" + count);
}
});
try {
Thread.sleep(1000); // 暂停一秒
} catch (InterruptedException e) {
e.printStackTrace();
}
count--;
}
// 倒计时结束
}
}).start();
```
3. 使用AsyncTask方式实现线程倒计时:
```
private class CountDownTask extends AsyncTask<Void, Integer, Void> {
@Override
protected Void doInBackground(Void... voids) {
int count = 10;
while (count >= 0) {
publishProgress(count);
try {
Thread.sleep(1000); // 暂停一秒
} catch (InterruptedException e) {
e.printStackTrace();
}
count--;
}
return null;
}
@Override
protected void onProgressUpdate(Integer... values) {
super.onProgressUpdate(values);
// 更新UI
tvCountDown.setText("" + values[0]);
}
@Override
protected void onPostExecute(Void aVoid) {
super.onPostExecute(aVoid);
// 倒计时结束
}
}
// 启动AsyncTask
new CountDownTask().execute();
```
需要注意的是,以上三种实现方式都有可能会出现卡顿的情况,特别是在倒计时时间较长的情况下。为了避免卡顿,可以考虑使用CountDownTimer类来实现线程倒计时。
相关推荐
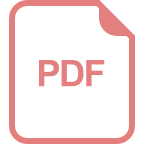
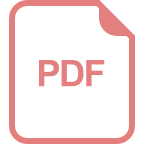














