时间换算,用结构类型表示时间内容,输入一个时间数,在输入一个秒数n,以h:m:s的格式输出过n秒后的时间值,用c语言写
时间: 2023-09-02 14:07:31 浏览: 43
可以使用结构体来表示时间,例如:
```c
struct Time {
int hour;
int minute;
int second;
};
```
然后可以编写一个函数来计算过了n秒后的时间值:
```c
#include <stdio.h>
struct Time {
int hour;
int minute;
int second;
};
struct Time addSeconds(struct Time t, int seconds) {
// 把秒数转换成小时、分钟、秒
int hours = seconds / 3600;
seconds -= hours * 3600;
int minutes = seconds / 60;
seconds -= minutes * 60;
// 把时间加上秒数
t.second += seconds;
if (t.second >= 60) {
t.second -= 60;
t.minute += 1;
}
t.minute += minutes;
if (t.minute >= 60) {
t.minute -= 60;
t.hour += 1;
}
t.hour += hours;
if (t.hour >= 24) {
t.hour -= 24;
}
return t;
}
int main() {
struct Time t = {10, 30, 0};
int n = 3600; // 假设过了1小时
struct Time newTime = addSeconds(t, n);
printf("%02d:%02d:%02d\n", newTime.hour, newTime.minute, newTime.second);
return 0;
}
```
这个程序会输出 `11:30:00`,表示过了1小时后的时间值。你可以根据需要修改输入的时间和秒数。
相关推荐
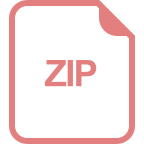
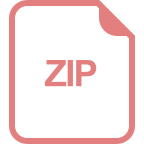
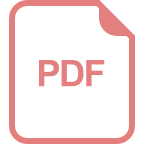












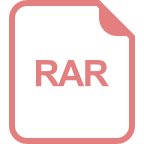
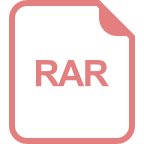