使用resnet骨干网络提取图像特征怎样加入inception多尺度模块
时间: 2023-08-04 22:04:28 浏览: 117
可以使用ResNet的最后一个卷积层的输出作为输入,然后添加Inception多尺度模块,将不同尺度的卷积核并行应用到输入特征上,最后将不同尺度的输出特征拼接在一起。具体步骤如下:
1. 使用ResNet骨干网络提取图像特征,得到最后一个卷积层的输出。
2. 定义Inception多尺度模块,包括不同尺度的卷积核和池化核,并行应用到输入特征上,得到不同尺度的输出特征。
3. 将不同尺度的输出特征拼接在一起,得到最终的特征表示。
4. 将最终的特征表示输入到全连接层进行分类或者回归等任务。
以下是代码示例,假设ResNet的最后一个卷积层输出为`x`,Inception多尺度模块包括3个分支:
```python
import torch.nn as nn
class InceptionModule(nn.Module):
def __init__(self, in_channels, out_channels):
super(InceptionModule, self).__init__()
# 1x1 convolution branch
self.branch1x1 = nn.Conv2d(in_channels, out_channels, kernel_size=1, stride=1)
# 3x3 convolution branch
self.branch3x3 = nn.Sequential(
nn.Conv2d(in_channels, out_channels, kernel_size=1, stride=1),
nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
)
# 5x5 convolution branch
self.branch5x5 = nn.Sequential(
nn.Conv2d(in_channels, out_channels, kernel_size=1, stride=1),
nn.Conv2d(out_channels, out_channels, kernel_size=5, stride=1, padding=2)
)
# max pooling branch
self.branch_pool = nn.Sequential(
nn.MaxPool2d(kernel_size=3, stride=1, padding=1),
nn.Conv2d(in_channels, out_channels, kernel_size=1, stride=1)
)
def forward(self, x):
out1x1 = self.branch1x1(x)
out3x3 = self.branch3x3(x)
out5x5 = self.branch5x5(x)
out_pool = self.branch_pool(x)
out = torch.cat([out1x1, out3x3, out5x5, out_pool], dim=1)
return out
class ResNetInception(nn.Module):
def __init__(self, num_classes):
super(ResNetInception, self).__init__()
self.resnet = models.resnet50(pretrained=True)
self.inception1 = InceptionModule(2048, 512)
self.inception2 = InceptionModule(2048 + 4 * 512, 1024)
self.inception3 = InceptionModule(2048 + 4 * 1024, 2048)
self.fc = nn.Linear(2048, num_classes)
def forward(self, x):
# ResNet backbone
x = self.resnet.conv1(x)
x = self.resnet.bn1(x)
x = self.resnet.relu(x)
x = self.resnet.maxpool(x)
x = self.resnet.layer1(x)
x = self.resnet.layer2(x)
x = self.resnet.layer3(x)
x = self.resnet.layer4(x)
# Inception module 1
out1 = self.inception1(x)
# Inception module 2
out2 = torch.cat([x, out1], dim=1)
out2 = self.inception2(out2)
# Inception module 3
out3 = torch.cat([x, out1, out2], dim=1)
out3 = self.inception3(out3)
# Global average pooling and fully connected layer
out = F.adaptive_avg_pool2d(out3, output_size=1)
out = out.view(out.size(0), -1)
out = self.fc(out)
return out
```
在上面的代码中,ResNet50骨干网络的输出为`x`,然后分别经过3个Inception多尺度模块,每个模块的输出作为下一个模块的输入。最终的特征表示为第3个模块的输出,经过全局平均池化后输入到全连接层进行分类。
阅读全文
相关推荐
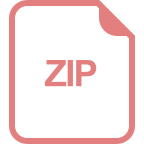

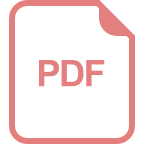
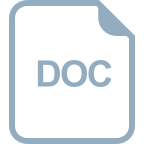
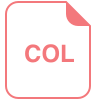
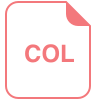
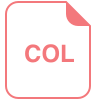
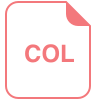

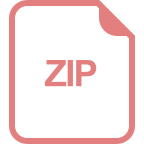
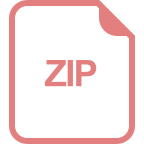
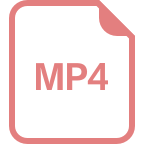
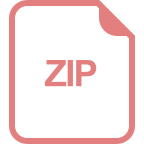
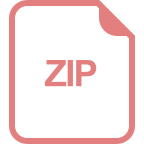
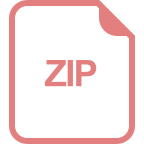