在matlab中使用resnet50骨干网络提取图像特征怎样加入inception多尺度模块
时间: 2023-12-03 19:06:23 浏览: 55
在 MATLAB 中使用 ResNet50 骨干网络提取图像特征,可以使用 `activations` 函数。这个函数可以把输入图像通过 ResNet50 模型进行前向传递,然后返回指定层的激活值,这些激活值可以作为特征向量来使用。
下面是一个示例代码:
```matlab
% Load the pre-trained ResNet50 network
net = resnet50;
% Load an example image
img = imread('example.jpg');
% Resize the image to fit the network input size
inputSize = net.Layers(1).InputSize;
img = imresize(img, [inputSize(1), inputSize(2)]);
% Extract features using the ResNet50 network
layer = 'fc1000';
features = activations(net, img, layer, 'OutputAs', 'rows');
```
这个代码会加载一个预训练的 ResNet50 网络,并读取一个示例图像 `example.jpg`。然后它将图像大小调整为网络的输入大小,最后通过网络的全连接层 `fc1000` 提取特征向量。
如果你想要在 ResNet50 的基础上添加 Inception 多尺度模块,可以参考 TensorFlow 中的实现方式。具体来说,你可以在 ResNet50 的最后一个卷积层后面添加 Inception 模块,然后再接一个全局池化层和一个全连接层,以输出特征向量。
在 MATLAB 中,你可以使用 `addLayers` 和 `connectLayers` 函数来添加新的层。下面是一个示例代码:
```matlab
% Define the Inception module
inceptionLayers = [
averagePooling2dLayer([5 5], 'Stride', 3, 'Name', 'inception_pool')
convolution2dLayer(1, 64, 'Name', 'inception_conv1x1')
reluLayer('Name', 'inception_relu1')
convolution2dLayer(3, 96, 'Padding', 'same', 'Name', 'inception_conv3x3_reduce')
reluLayer('Name', 'inception_relu2')
convolution2dLayer(3, 128, 'Padding', 'same', 'Name', 'inception_conv3x3')
reluLayer('Name', 'inception_relu3')
convolution2dLayer(1, 32, 'Name', 'inception_conv5x5_reduce')
reluLayer('Name', 'inception_relu4')
convolution2dLayer(5, 64, 'Padding', 'same', 'Name', 'inception_conv5x5')
reluLayer('Name', 'inception_relu5')
concatenationLayer(4, 3, 'Name', 'inception_concat')
];
% Load the pre-trained ResNet50 network
net = resnet50;
% Remove the last two layers (average pooling and fc1000)
layersToRemove = {'avg_pool', 'fc1000'};
net = removeLayers(net, layersToRemove);
% Add the Inception module after the last conv layer
lastConvLayer = findLastConvLayer(net);
net = addLayers(net, inceptionLayers);
net = connectLayers(net, lastConvLayer, 'inception_pool');
% Add a global pooling layer and a fully connected layer
net = globalAveragePooling2dLayer('Name', 'global_pool')(net);
net = fullyConnectedLayer(1024, 'Name', 'fc1024')(net);
net = reluLayer('Name', 'relu')(net);
% Extract features using the modified network
img = imread('example.jpg');
inputSize = net.Layers(1).InputSize;
img = imresize(img, [inputSize(1), inputSize(2)]);
layer = 'fc1024';
features = activations(net, img, layer, 'OutputAs', 'rows');
```
这个代码首先定义了一个 Inception 模块 `inceptionLayers`。然后它加载一个预训练的 ResNet50 网络,并移除最后两层。接着它找到最后一个卷积层,并在其后面添加 Inception 模块。最后它再添加一个全局池化层和一个全连接层,以输出特征向量。
相关推荐
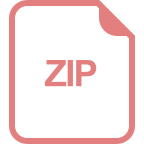
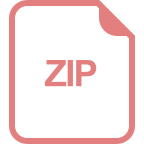
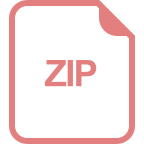














