使用vue计算多名同学的某一门课程的平均值
时间: 2023-06-13 22:03:32 浏览: 102
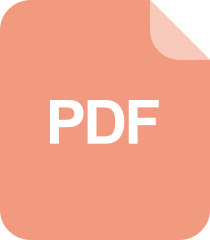
vue计算属性及使用详解

假设你有一个包含学生信息的数组,每个学生信息包含学生的姓名和成绩。你可以使用Vue的计算属性来计算多名同学的某一门课程的平均值。以下是一个示例:
```html
<template>
<div>
<h2>学生成绩列表</h2>
<table>
<thead>
<tr>
<th>姓名</th>
<th>语文成绩</th>
<th>数学成绩</th>
<th>英语成绩</th>
</tr>
</thead>
<tbody>
<tr v-for="student in students" :key="student.name">
<td>{{ student.name }}</td>
<td>{{ student.chinese }}</td>
<td>{{ student.math }}</td>
<td>{{ student.english }}</td>
</tr>
<tr>
<td>平均分</td>
<td>{{ chineseAverage }}</td>
<td>{{ mathAverage }}</td>
<td>{{ englishAverage }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
students: [
{ name: '张三', chinese: 80, math: 90, english: 85 },
{ name: '李四', chinese: 75, math: 85, english: 80 },
{ name: '王五', chinese: 90, math: 95, english: 90 },
],
};
},
computed: {
chineseAverage() {
const sum = this.students.reduce((acc, cur) => acc + cur.chinese, 0);
return (sum / this.students.length).toFixed(2);
},
mathAverage() {
const sum = this.students.reduce((acc, cur) => acc + cur.math, 0);
return (sum / this.students.length).toFixed(2);
},
englishAverage() {
const sum = this.students.reduce((acc, cur) => acc + cur.english, 0);
return (sum / this.students.length).toFixed(2);
},
},
};
</script>
```
在这个示例中,我们首先定义了一个包含学生信息的数组`students`。然后,我们使用Vue的计算属性来计算每门课程的平均值。在`chineseAverage`计算属性中,我们使用`reduce`方法来计算所有学生的语文成绩之和,然后除以学生总数来计算平均值。最后,我们使用`toFixed`方法来保留两位小数。其他两个计算属性也是类似的。最终,我们在表格中显示每个学生的成绩,并在表格底部显示每门课程的平均值。
阅读全文
相关推荐
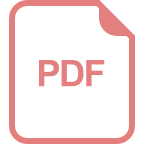

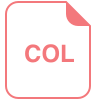


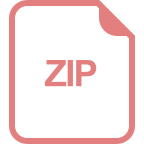
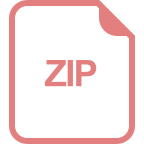
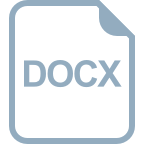
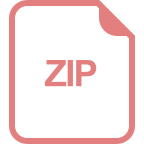
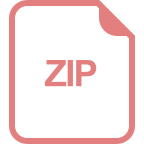