import os # 输入文件名、新 ID 文件名和输出文件名 input_file = "example.fasta" new_id_file = "new_ids.txt" output_file = "example_new.fasta" # 检查输入文件、新 ID 文件和输出文件是否存在 if not os.path.exists(input_file): print("Error: Input file does not exist!") exit() if not os.path.exists(new_id_file): print("Error: New ID file does not exist!") exit() # 读取新 ID 文件中的内容 new_ids = {} with open(new_id_file, "r") as f: for line in f: old_id, new_content = line.strip().split() new_ids[old_id] = new_content # 打开输入文件和输出文件 with open(input_file, "r") as f_in, open(output_file, "w") as f_out: # 初始化变量 current_id = "" current_seq = "" # 读取输入文件的每一行 for line in f_in: # 如果这一行是 ID 行,则保存上一个序列并更新当前 ID if line.startswith(">"): if current_id: new_content = new_ids.get(current_id, "") f_out.write(current_id + new_content + "\n") f_out.write(current_seq + "\n") current_id = line.strip() current_seq = "" # 如果这一行是序列行,则将序列加入当前序列中 else: current_seq += line.strip() # 保存最后一个序列 if current_id: new_content = new_ids.get(current_id, "") f_out.write(current_id + new_content + "\n") f_out.write(current_seq + "\n") print("Done!")ValueError: too many values to unpack (expected 2)
时间: 2024-03-28 15:36:51 浏览: 14
这段代码是用 Python 编写的文件处理程序,用于将一个 FASTA 格式文件中的 ID 进行替换,并输出新的 FASTA 格式文件。其中,输入文件名、新 ID 文件名和输出文件名分别为 input_file、new_id_file 和 output_file。程序的主要逻辑是,逐行读取输入文件,判断当前行是否是 ID 行,如果是则保存上一个序列并更新当前 ID,如果不是,则将当前行的序列加入当前序列中。同时,程序通过读取新 ID 文件中的内容,将旧的 ID 替换为新的 ID,并写入新的 FASTA 格式文件中。
这段代码在读取新 ID 文件时,使用 strip() 和 split() 方法分别去除行末的空白符并以空格为分隔符将一行文本拆分成两个部分,分别赋值给 old_id 和 new_content。但是,如果一行文本中包含多个空格,则会出现“too many values to unpack”异常。这个错误的原因是 split() 方法返回的列表中元素的数量超过了变量的数量。可能的解决方法是,使用 rsplit() 方法,并设置分隔符的最大分割次数为 1,即:
old_id, new_content = line.strip().rsplit(" ", 1)
相关问题
import sys# 从命令行获取fasta文件名和新id列表文件名fasta_file = sys.argv[1]id_file = sys.argv[2]# 读取新id列表new_ids = []with open(id_file, 'r') as f: for line in f: new_ids.append(line.strip())# 打开fasta文件,读取并修改idwith open(fasta_file, 'r') as f: for line in f: if line.startswith('>'): # 获取旧id old_id = line.strip() # 获取新id new_id = new_ids.pop(0) # 输出新id print(new_id) else: # 输出序列信息 print(line.strip())
这是一个Python脚本,用于从命令行获取一个fasta文件和一个新id列表文件的名称,并根据新id列表文件中的id替换fasta文件中的旧id。
脚本的主要步骤如下:
1. 导入sys模块,以获取命令行参数。
2. 从命令行获取fasta文件名和新id列表文件名。
3. 读取新id列表文件,并将每个新id添加到一个列表中。
4. 打开fasta文件,逐行读取文件内容。
5. 如果读取的行以“>”开头,则表示这是一个id行,需要替换旧id为新id。
6. 从新id列表中弹出第一个id,并将其作为新id。
7. 输出新id。
8. 如果读取的行不是id行,则表示这是序列信息,直接输出。
9. 循环处理fasta文件中的所有行。
请注意,此脚本仅输出替换后的fasta文件内容,而没有将其写入到任何文件中。如果需要将其写入文件,需要在脚本中添加文件写入代码。
报错new_id = new_ids.pop(0) IndexError: pop from empty list
这个错误提示说明new_ids这个列表为空,而你的代码又尝试从它的开头取出一个元素,所以导致了IndexError异常。这可能是因为id_file文件为空或者文件中的行数少于fasta_file中的序列数。
为了避免这个错误,你可以在读取完id_file文件之后,先检查一下new_ids列表的长度,如果是0,则立即退出循环。修改后的代码如下所示:
```python
import sys
def replace_fasta_ids(fasta_file, id_file):
# 读取新id列表
with open(id_file, 'r') as f:
new_ids = [line.strip() for line in f]
# 替换fasta文件中的id
new_fasta = ''
with open(fasta_file, 'r') as f:
for line in f:
if line.startswith('>'):
# 获取旧id
old_id = line.strip()
if len(new_ids) == 0: # 检查new_ids列表是否为空
break
# 获取新id
new_id = new_ids.pop(0)
# 替换id
new_fasta += '>' + new_id + '\n'
else:
# 添加序列信息
new_fasta += line
# 将替换后的fasta写入文件
with open('new.fasta', 'w') as f:
f.write(new_fasta)
return new_fasta
if __name__ == '__main__':
if len(sys.argv) != 3:
print('Usage: python script.py fasta_file id_file')
sys.exit(1)
fasta_file = sys.argv[1]
id_file = sys.argv[2]
try:
replace_fasta_ids(fasta_file, id_file)
except Exception as e:
print('Error:', e)
sys.exit(1)
```
这样修改之后,如果new_ids列表为空,程序会立即退出循环,不会再尝试取出元素,就不会出现IndexError异常了。
相关推荐
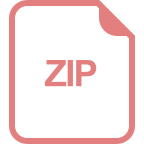
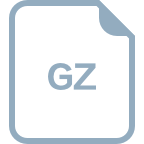






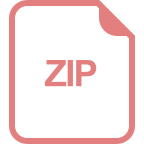
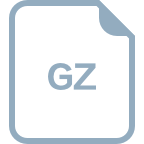
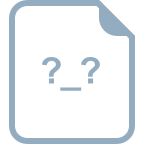
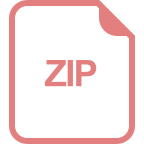