用C语言编写文件复制程序。分别使用系统调用read (fd,buf,nbytes)、write(fd, buf,nbytes)和文件的库函数fread(buf,size,nitems,fp)、fwrite(buf,size,nitems,fp),编写一个文件复制程序。
时间: 2024-02-03 21:15:08 浏览: 54
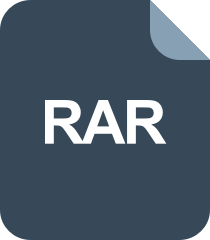
利用汇编写的文件操作小程序,功能是复制文件
使用系统调用read和write函数的文件复制程序:
```c
#include <unistd.h>
#include <fcntl.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
int source_file = open(argv[1], O_RDONLY);
if (source_file == -1) {
perror("Failed to open source file");
return 1;
}
int dest_file = open(argv[2], O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (dest_file == -1) {
perror("Failed to create destination file");
return 1;
}
char buffer[BUFFER_SIZE];
ssize_t read_bytes;
while ((read_bytes = read(source_file, buffer, BUFFER_SIZE)) > 0) {
ssize_t written_bytes = write(dest_file, buffer, read_bytes);
if (written_bytes != read_bytes) {
perror("Failed to write to destination file");
return 1;
}
}
if (read_bytes < 0) {
perror("Failed to read from source file");
return 1;
}
close(source_file);
close(dest_file);
return 0;
}
```
使用文件的库函数fread和fwrite函数的文件复制程序:
```c
#include <stdio.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
FILE *source_file = fopen(argv[1], "rb");
if (source_file == NULL) {
perror("Failed to open source file");
return 1;
}
FILE *dest_file = fopen(argv[2], "wb");
if (dest_file == NULL) {
perror("Failed to create destination file");
return 1;
}
char buffer[BUFFER_SIZE];
size_t read_items;
while ((read_items = fread(buffer, 1, BUFFER_SIZE, source_file)) > 0) {
size_t written_items = fwrite(buffer, 1, read_items, dest_file);
if (written_items != read_items) {
perror("Failed to write to destination file");
return 1;
}
}
if (ferror(source_file)) {
perror("Failed to read from source file");
return 1;
}
fclose(source_file);
fclose(dest_file);
return 0;
}
```
阅读全文
相关推荐
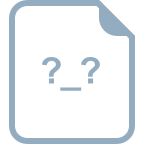







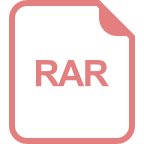
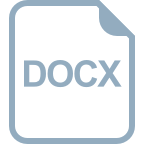