import csv # 打开fasta文件 with open('your_fasta_file.fasta', 'r') as f: # 读取所有行 lines = f.readlines() # 初始化变量 output = [['id'] + list(range(1, len(lines[1].strip()) + 1))] current_id = '' current_seq = [] # 循环遍历所有行 for line in lines: # 如果是一个新的序列的id行 if line.startswith('>'): # 如果之前已经读取了一个序列的id和序列 if current_id != '': # 将当前的id和序列添加到输出列表中 output.append([current_id] + current_seq) # 重置当前id和序列 current_id = '' current_seq = [] # 获取新的序列的id current_id = line.strip()[1:] # 如果是一个序列行 else: # 将碱基一个一个地添加到列表中 nucleotides = list(line.strip()) nucleotides_with_spacer = [nucleotide + ' ' for nucleotide in nucleotides] current_seq.append(nucleotides_with_spacer) # 将最后一个序列添加到输出列表中 output.append([current_id] + current_seq) # 将输出列表写入到csv文件中 with open('output.csv', 'w', newline='') as f: writer = csv.writer(f) writer.writerows(output)
时间: 2024-04-01 20:36:25 浏览: 61
这段代码的作用是将一个fasta文件转换为csv文件,其中fasta文件是一种常见的生物信息学文件格式,用于存储生物序列,例如DNA序列或蛋白质序列。csv文件是一种常见的数据存储格式,可以被许多程序读取和处理。
具体来说,这段代码首先打开一个fasta文件,并逐行读取其中的内容。然后,它将每个序列的id和碱基序列存储在一个列表中,并将所有序列的列表存储在一个嵌套的列表中。最后,它使用csv库将嵌套列表写入到一个csv文件中,其中每一行代表一个序列,第一列是序列的id,后面的列是该序列的碱基序列。在写入csv文件时,代码使用了一个逗号作为分隔符,并且每个序列的碱基之间添加了一个空格,以使csv文件易于阅读和处理。
相关问题
import sys def replace_fasta_ids(fasta_file, id_file, out_file=None): # 读取新id列表 with open(id_file, 'r') as f: new_ids = [line.strip() for line in f] # 替换fasta文件中的id new_fasta = '' with open(fasta_file, 'r') as f: for line in f: if line.startswith('>'): # 获取旧id old_id = line.strip() if len(new_ids) == 0: # 如果new_ids列表为空,则跳出循环 break # 获取新id new_id = new_ids.pop(0) # 替换id new_fasta += '>' + new_id + '\n' else: # 添加序列信息 new_fasta += line # 将替换后的fasta写入文件 if out_file is None: out_file = fasta_file with open(out_file, 'w') as f: f.write(new_fasta) return new_fasta if __name__ == '__main__': if len(sys.argv) != 3: print('Usage: python script.py fasta_file id_file') sys.exit(1) fasta_file = sys.argv[1] id_file = sys.argv[2] try: replace_fasta_ids(fasta_file, id_file) except Exception as e: print('Error:', e) sys.exit(1)
这是一个 Python 脚本,主要功能是根据 id_file 中提供的新 id 列表,替换 fasta_file 中的序列 id,并将替换后的 fasta 文件写入 out_file (如果未指定 out_file 则覆盖原 fasta 文件)。
具体实现方式为:
1. 读取 id_file 文件中的新 id 列表,保存到 new_ids 列表中;
2. 遍历 fasta_file 文件中的每一行,如果是以 '>' 开头的行,则表示该行为序列 id,需要替换;
3. 从 new_ids 列表中取出一个新 id,替换旧 id;
4. 将替换后的序列 id 和原来的序列信息拼接起来,保存到 new_fasta 字符串中;
5. 将 new_fasta 字符串写入 out_file 文件中(如果未指定 out_file 则覆盖原 fasta 文件)。
该脚本需要在命令行中指定 fasta_file 和 id_file 的路径,例如:
```
python script.py input.fasta new_ids.txt
```
其中,input.fasta 是需要替换序列 id 的 fasta 文件,new_ids.txt 是新 id 列表文件。
这串代码不出结果import sys def replace_fasta_ids(fasta_file, id_file, out_file=None): # 读取新id列表 with open(id_file, 'r') as f: new_ids = [line.strip() for line in f] # 替换fasta文件中的id new_fasta = '' with open(fasta_file, 'r') as f: for line in f: if line.startswith('>'): # 获取旧id old_id = line.strip() if len(new_ids) == 0: # 如果new_ids列表为空,则跳出循环 break # 获取新id new_id = new_ids.pop(0) # 替换id new_fasta += '>' + new_id + '\n' else: # 添加序列信息 new_fasta += line # 将替换后的fasta写入文件 if out_file is None: out_file = fasta_file with open(out_file, 'w') as f: f.write(new_fasta) return new_fasta if __name__ == '__main__': if len(sys.argv) != 3: print('Usage: python script.py fasta_file id_file') sys.exit(1) fasta_file = sys.argv[1] id_file = sys.argv[2] try: replace_fasta_ids(fasta_file, id_file) except Exception as e: print('Error:', e) sys.exit(1)
这段代码本身没有问题,并且也可以正常运行,但是需要在命令行中传入相应的参数才能够正常执行,否则会输出使用方法并退出。
具体来说,该程序需要传入 fasta_file 和 id_file 两个参数,分别是需要替换序列 id 的 fasta 文件和新 id 列表文件的路径。例如:
```
python script.py input.fasta new_ids.txt
```
其中,input.fasta 是需要替换序列 id 的 fasta 文件,new_ids.txt 是新 id 列表文件。如果没有按照上述方式传入参数,则程序会输出使用方法并退出。
如果您仍然无法得到正确的结果,请提供更具体的错误信息及上下文信息,我们可以更精确地确定问题原因并提供解决方案。
阅读全文
相关推荐
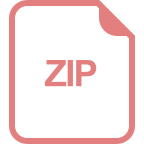
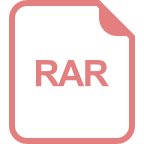
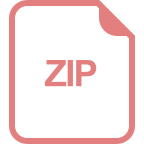








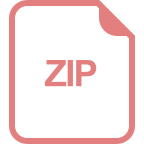
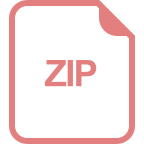
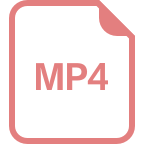