如何用Python实现一个函数,从单链表中删除最大值节点并返回该最大值?同时,请提供相关的代码示例。
时间: 2024-11-04 11:11:15 浏览: 8
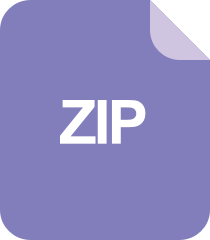
getMaxMises_pythonabaqus_abaqus最大值_python_abaqus最大_

在Python中,我们可以首先创建一个Node类表示链表的节点,然后定义一个LinkedList类来存储链表,并实现删除最大值节点的功能。以下是代码实现:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
# 添加节点到链表末尾
def append(self, data):
if not self.head:
self.head = Node(data)
else:
current = self.head
while current.next:
current = current.next
current.next = Node(data)
# 删除最大值节点
def delete_max(self):
if not self.head or not self.head.next:
return None, self.head.data if self.head else None # 如果链表为空或只有一个元素,直接返回头节点及其值
max_value = self.head.data # 初始化最大值为头节点的值
max_node = self.head # 记录最大值所在节点
current = self.head.next # 遍历链表
while current:
if current.data > max_value:
max_value = current.data
max_node = current
current = current.next
# 删除最大值节点
if max_node != self.head: # 如果最大值节点不是头节点
max_node.prev.next = max_node.next
else: # 如果最大值节点是头节点
self.head = max_node.next if max_node.next else None
return max_node, max_value
# 示例:
ll = LinkedList()
ll.append(5)
ll.append(10)
ll.append(3)
max_node, max_value = ll.delete_max()
print("删除最大值后的链表:", ll)
print("最大值节点和值:", max_node, max_value) # 输出:(10, 10)
```
在这个例子中,我们首先遍历链表找到最大值节点,然后更新其前驱节点的next指针将其删除。
阅读全文
相关推荐
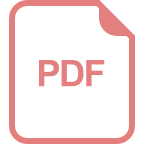
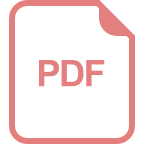















